Understanding Variables in PHP: A Beginner’s Guide
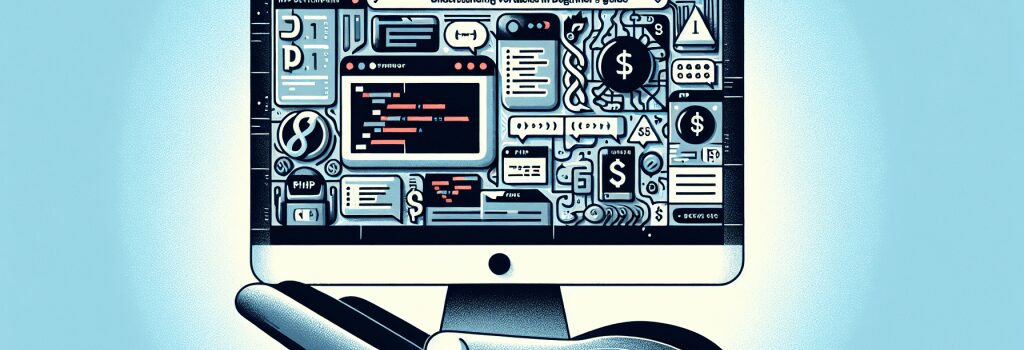
Introduction to PHP Variables
Variables are essential components in any programming language. They act as containers for storing data values. In PHP, a server-side scripting language designed primarily for web development, understanding how to effectively work with variables is crucial for beginners. This guide will introduce you to the basics of PHP variables, their significance, and how to use them in your web development journey.
What are Variables in PHP?
In PHP, a variable starts with a dollar sign ($) followed by the name of the variable. The name of the variable can start with a letter or an underscore (not a number), followed by any number of letters, numbers, or underscores. Variables in PHP are case-sensitive, meaning ;$Variable>, ;$VARIABLE>, and ;$variable> are considered three different variables.
Declaring Variables in PHP
To declare a variable in PHP, you simply need to write the dollar sign followed by the name of the variable and assign a value to it using the equals sign (;=>). PHP is a loosely typed language, which means you do not need to declare a variable’s type. Based on the assigned value, PHP automatically converts the variable into its appropriate data type.
In the example above, ;$greeting> is a string, ;$year> is an integer, and ;$isLearningFun> is a boolean.
Understanding Data Types in PHP
PHP supports several data types that you can assign to variables. These include:
– Strings: A sequence of characters, used for textual content.
– Integers: Whole numbers, without a decimal point.
– Floats (also known as doubles): Numbers with a decimal point or numbers in exponential form.
– Booleans: Represents two states, ;TRUE> or ;FALSE>.
– Arrays: Holds multiple values in a single variable.
– Objects: Instances of classes, used in object-oriented programming.
– NULL: Represents a variable with no value.
Operators in PHP
PHP provides a wide range of operators to perform operations on variables and their values. These include arithmetic operators, assignment operators, comparison operators, increment/decrement operators, logical operators, and string operators.
How to Use Variables in PHP
Variables in PHP can be used in various ways. They can store user inputs, calculate values, hold database query results, and much more. Here’s an example of how variables can be used in a simple PHP program:
Best Practices for Working with Variables in PHP
– Naming Conventions: Use meaningful variable names that describe the contained values. This improves code readability and maintainability.
– Avoid Global Variables: Minimize the use of global variables as they can lead to code that is difficult to debug and maintain.
– Keep Variable Types in Mind: Even though PHP is a loosely typed language, being mindful of data types can prevent unexpected behavior and errors in your code.
Conclusion
Variables are the foundational elements of any PHP program. As you start your journey into web development with PHP, mastering the use of variables, understanding their data types, and applying operators will allow you to manipulate data effectively and create dynamic web applications. Remember, practice makes perfect. So, experiment with variables as much as you can and observe how they behave in different scenarios to deepen your understanding of PHP programming.