Overcoming Common Debugging Challenges in Vue.js for Web Development
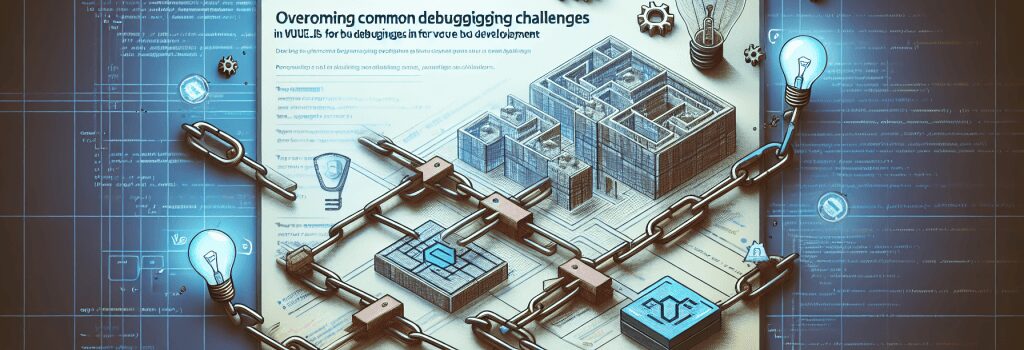
So, you’ve decided to become a web developer and now you’re knee-deep in coding languages like HTML, PHP, CSS, JavaScript, and WordPress. That’s pretty impressive, but we’ve got one more challenge to throw at you – Vue.js. And it’s not just about understanding Vue.js, it’s about overcoming common debugging challenges in Vue.js for web development. Sounds quite daunting, doesn’t it? Don’t worry, we’ve got you covered.
What is Vue.js?
Before we learn how to overcome debugging challenges, let’s quickly define what Vue.js is. It’s a progressive framework for building user interfaces. It’s like that interactive, multi-layered cake where if you poke one layer, the others react accordingly. Fancy, right? However, just like any other delicacy, it can sometimes have some hidden nuts or, in developer language, bugs.The Common Debugging Challenges in Vue.js
Like any good detective, the first step to solving a problem is identifying it. Here are some common Vue.js debugging challenges:1. Data not being reactive: This is like playing a game of Whac-A-Mole. You change the data in one place and expect it to reflect somewhere else, but it just refuses to budge.
2. Misuse of Vue.js directives: Directives are like the gossip aunties of a neighborhood. They keep an eye on everything and, if used incorrectly, they can create quite a ruckus.
3. Component not rendering or not updating: This is like talking to a wall. You keep feeding in commands, but the component just doesn’t respond.
Overcoming Vue.js Debugging challenges
Now let’s put on our superhero coder capes and find out how to fight these villains!Making Data Reactive
Remember our good old friend, the Whac-A-Mole game? To win, we have to ensure that each change in data is properly watched by Vue.js. How do we do that? By declaring all the data properties upfront in the data option.Proper Use of Vue.js Directives
Keep the gossip aunties happy by using Vue.js directives like v-if, v-else, v-show, v-on, v-bind correctly. Each has its own special function and using them in the right place at the right time can avoid many unnecessary bugs.Components Rendering or Updating
Now to avoid talking to the wall, always remember to use the “key” attribute when using v-for directive to iterate through arrays or objects. This helps Vue.js keep track of each node’s identity and it sure helps in rendering or updating the component correctly.In conclusion, as a budding web developer, you’ll come across many challenges. Remember, every superhero needs a challenge to prove their superpowers. Vue.js debugging might seem like a formidable foe, but with the right knowledge, you’ll transform from being a coder in distress to a debugging superhero. Don’t forget, every bug fixed is a step closer to becoming a web development maven! Keep an eye out for more helpful resources in your quest for developing mastery.
FAQ
How do I troubleshoot a Vue.js app that is not displaying any content?
Sometimes, this can be caused by incorrect syntax or missing data bindings in your Vue components. Check the console for any error messages, inspect the HTML elements in the browser’s developer tools, and make sure your data is properly passed to the components.
Why is my Vue.js app not updating when I change the data?
This could be due to improperly using reactivity in Vue. Ensure that you are updating the data properties correctly using methods like `this.$set` or `Object.assign` for reactive objects.
How do I handle errors and exceptions in Vue.js?
Vue provides error handling mechanisms like the `ErrorCaptured` hook and `errorCaptured` event to handle errors at the component level. You can also use `try-catch` blocks in methods to catch errors.
Why is my event handling not working in Vue.js?
Double-check your event bindings and make sure that the event listeners are correctly set up. Also, verify the event propagation and check if any other elements are intercepting the events.
Why are my computed properties not updating in Vue.js?
Computed properties depend on their dependencies being updated. If your computed property is not updating, check if the dependent data properties are changing and that you are not mutating them directly.
How can I optimize the performance of my Vue.js app?
Use `v-if` and `v-show` directives strategically to reduce unnecessary rendering, implement virtual scrolling for large lists, and lazy-load components or assets to improve the initial loading time.
How do I debug Vue.js applications in the browser?
Utilize the Vue.js DevTools browser extension to inspect Vue components, data, events, and performance metrics. You can also use `console.log` statements and breakpoints in the browser’s developer tools for debugging.
Is it possible to use third-party libraries and plugins in Vue.js?
Yes, Vue.js provides a way to integrate third-party libraries and plugins using `Vue.use()` or by registering them as global components. Make sure to follow the documentation and best practices for each specific library or plugin.
How can I optimize the bundle size of my Vue.js application?
Consider using code-splitting techniques to load components dynamically, analyze and remove unused dependencies with tools like Webpack Bundle Analyzer, and implement tree shaking to eliminate dead code during the build process.
What are some common pitfalls to avoid when developing with Vue.js?
Avoid mutating data directly, using `v-for` with `v-if` on the same element, forgetting to provide unique `key` attributes in list rendering, and relying too heavily on watchers instead of computed properties when unnecessary.