Implementing Mocks and Stubs in PHP Unit Testing
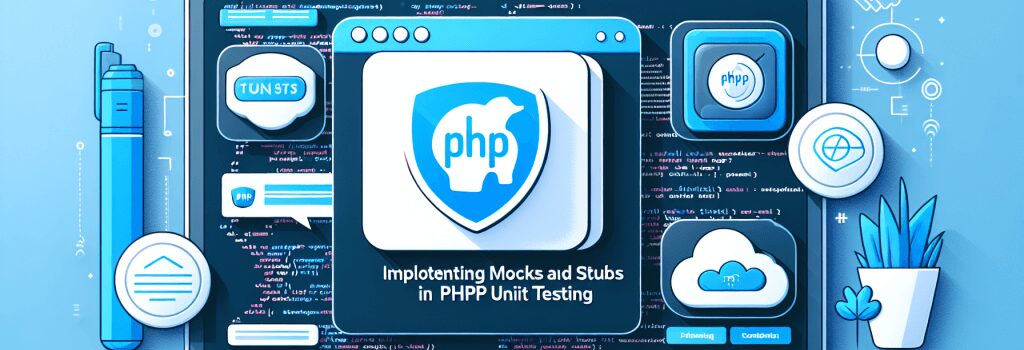
Welcome, budding coders, to the fantastical world of web development! Today we’re diving headfirst into the rabbit hole of PHP Unit Testing, focusing primarily on the art of implementing Mocks and Stubs. Now, strap on your helmets, because it’s about to get geeky!
Before we plunge into the deep end though, let’s ensure we’re all singing from the same hymn sheet. Unit Testing, in the simplest sense, is a way of checking that individual components of your code are working as intended. Imagine it like a movie rehearsal before the big shoot. Mocks and Stubs are our superstar stand-ins, helping us simulate certain conditions and responses for a smoother rehearsal.
What are Mocks and Stubs?
Let’s clarify these terms before we move forward. In the PHP unit testing universe, a ‘Mock’ is an imitation of a real object, with the ability to decide which methods can be invoked. If your code was a puppet show, Mocks would be the puppet masters.
‘Stubs’ on the other hand, aren’t quite as controlling. They’re more like the understudies, with pre-programmed responses, stepping in for the real deal when needed.
The Beauty of Mocks and Stubs
Mocks and Stubs are like superheroes, ready to clean up Gotham or well, your code. They isolate your testing environment, helping you avoid any real-world consequences. If something goes south, the real methods and databases remain unscathed, and you, dear coder, can fix the damage behind the scenes. It’s like having a code safety net, allowing you to perform daring acrobatics without worrying about a high-level fallout.
Implementing Mocks and Stubs
The Adventure Begins with PHPUnit
PHP Unit Testing uses PHPUnit, a framework that makes testing more efficient. But enough chit-chat, let’s dig into some pseudo-code examples.
Mocking Around
Creating a Mock is as simple as this:
We’ve just created a mock object of ClassToMock. We can then further define what functions we want our Mock to copycat.
Stubbing our Journey
Creating a Stub is not much different:
The above stub will always return ‘Hey, I am a stub!’ when someMethod is invoked. Talk about an overachiever!
Handling Exceptions
The mocking and stubbing game can be tricky when exceptions enter the picture. Fortunately, PHPUnit provides a way to handle these:
This sets our Stub so that calling the exceptionThrowingMethod now results in an exception. It’s like provoking a virtual bull in a safety-confined arena.
Voilà! We’ve just tackled the basics of implementing Mocks and Stubs in PHP Unit Testing. Don’t forget to practice, because coding, like telling a good joke, always gets better with repetition!
Conquering Mocks and Stubs is a badge of honor in your coding journey, so pat yourself on the back and revel in your newfound knowledge. Remember, in the world of coding, there is no ‘Mission Impossible’. With enough persistence and cheeky humor, you’re on your way to creating the most resilient, robust, and frankly, fun code out there. Happy coding!