The Essential Guide to PHP Arithmetic and Comparison Operators
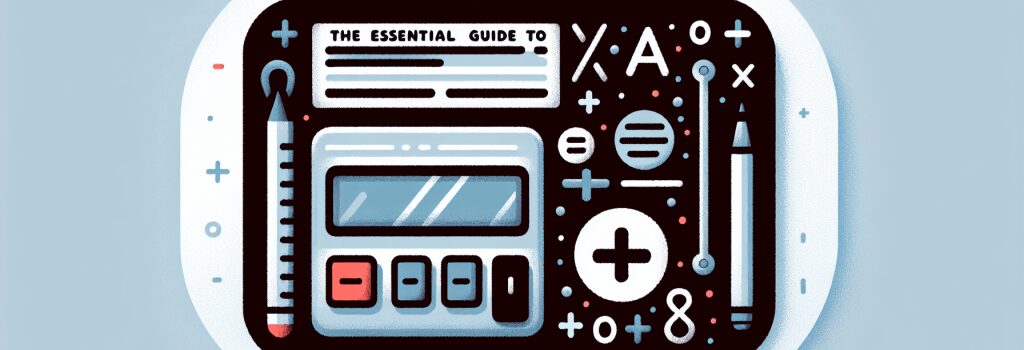
Understanding PHP Arithmetic and Comparison Operators: A Comprehensive Guide
Introduction to PHP Operators
PHP, a server-side scripting language, is essential for web developers looking to build dynamic and interactive websites. A fundamental aspect of PHP, and programming in general, involves using operators to manipulate data and variables. This guide focuses on two critical types of operators in PHP: arithmetic and comparison operators. Mastering these operators is crucial for performing calculations, evaluating conditions, and making decisions in your PHP scripts.
Arithmetic Operators in PHP
Arithmetic operators are symbols that perform mathematical operations on numerical values. PHP supports several arithmetic operators, making it straightforward to execute basic mathematical operations.
Addition (;+>)
The addition operator adds two operands. For example, ;$x + $y> will sum the values of ;$x> and ;$y>.
Subtraction (;->)
This operator subtracts the second operand from the first. So, ;$x – $y> will subtract ;$y> from ;$x>.
Multiplication (;*>)
Multiplication uses the asterisk symbol to multiply two operands, as in ;$x * $y>.
Division (;/>)
The division operator divides the first operand by the second, indicated by ;$x / $y>.
Modulus (;%>)
Modulus returns the remainder of dividing the first operand by the second. It’s represented by ;$x % $y>.
Exponentiation (;<strong></strong>>)
This operator raises the first operand to the power of the second, as in ;$x $y>, where ;$x> is the base, and ;$y> is the exponent.
Comparison Operators in PHP
Comparison operators compare two values and return a boolean value: ;true> or ;false>. These operators are crucial for making decisions and controlling the flow of the program.
Equal (;==>)
This operator checks if two values are equal, disregarding their data type. For example, ;5 == “5”> evaluates to ;true>.
Identical (;===>)
The identical operator also checks equality but considers the data type. Thus, ;5 === “5”> is ;false>.
Not Equal (;!=> or ;>)
These operators check if two values are not equal. Both ;5 != “5”> and ;5 “5”> yield ;false> because it only looks at the value.
Not Identical (;!==>)
It verifies that two values are not equal or they are not of the same type. Therefore, ;5 !== “5”> is ;true>.
Greater Than (;>>)
Checks if the value on the left is greater than the value on the right.
Less Than (;<>)
Determines if the value on the left is less than the value on the right.
Greater Than or Equal To (;>=>)
This operator checks if the left operand is greater than or equal to the right operand.
Less Than or Equal To (;<=>)
It verifies if the left operand is less than or equal to the right operand.
Conclusion
In PHP development, understanding and applying arithmetic and comparison operators are foundational skills. These operators allow you to perform mathematical operations and make logical decisions based on the comparison of values. By mastering these operators, you will enhance your capability to develop complex and logic-driven web applications. Remember, the key to becoming proficient in PHP, or any programming language, lies in practicing and applying these concepts in real-world projects.
By learning to effectively use arithmetic and comparison operators, you’re taking significant steps towards becoming a skilled web developer. Keep experimenting with these operators in different scenarios to reinforce your understanding and discover more about PHP’s powerful capabilities.