Operators in JavaScript: Making Sense of Symbols
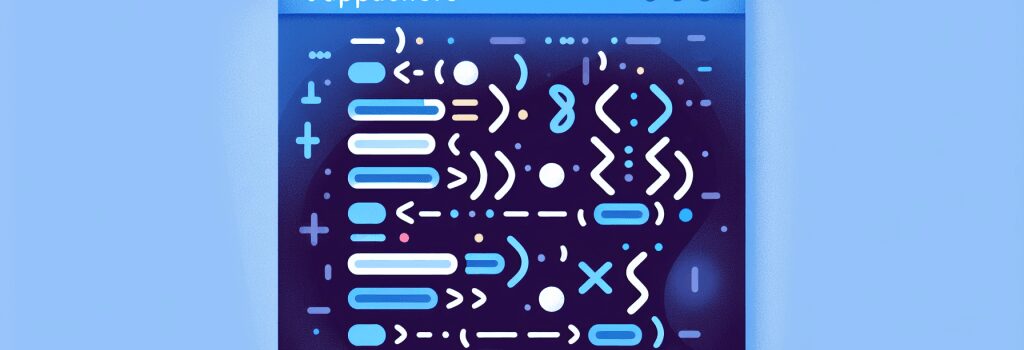
Operators in JavaScript: Making Sense of Symbols
JavaScript operators play a critical role in scripting dynamic behaviors and interactions on the web. They are symbols that operate on one or more operands (values or variables) to perform a variety of operations, encompassing everything from basic arithmetic to complex logical evaluations. Below, we break down these operators into understandable segments to empower your journey in becoming a proficient web developer.
Understanding JavaScript Operators
Operators in JavaScript can be primarily categorized into several types, each serving distinct purposes in your code. By mastering these, you can manipulate data and logic within your applications effectively.
Arithmetic Operators
Arithmetic operators allow the performance of mathematical calculations. These include:
– ;+> (Addition): Combines two values.
– ;-> (Subtraction): Finds the difference between values.
– ;> (Multiplication): Calculates the product.
– ;/> (Division): Divides the first operand by the second.
– ;%> (Modulus): Returns the division remainder.
– ;++> (Increment): Increases a value by one.
– ;–> (Decrement): Reduces a value by one.
Assignment Operators
Assignment operators are used to assign values to JavaScript variables. The most common example is the ;=> operator, but there are others like ;+=>, ;-=>, ;=>, and ;/=> that combine assignment with arithmetic operations.
Comparison Operators
To compare two values, JavaScript offers comparison operators which are critical in control flow statements like if-else conditions. Examples include:
– ;==> (Equal to): Checks if two values are equal.
– ;===> (Strictly equal to): Same as above but also compares data types.
– ;!=> (Not equal to): Tests if values are not equal.
– ;>> (Greater than), ;=> (Greater than or equal to), ;<=> (Less than or equal to): These allow comparison of values in terms of magnitude.
Logical Operators
Logical operators are utilized in decision making and are especially useful in evaluating multiple conditions. They include:
– ;&&> (Logical AND): Returns true if both operands are true.
– ;||> (Logical OR): True if either operand is true.
– ;!> (Logical NOT): Inverts the truthiness of the operand.
String Operators
In JavaScript, ;+> can also serve as a concatenation operator when used with strings, merging them into one.
Conditional (Ternary) Operator
The ternary operator is the only JavaScript operator that takes three operands, typically used as a shortcut for an if-else statement. It is denoted as ;condition ? valueIfTrue : valueIfFalse>.
Best Practices
– Understand operator precedence: Knowing which operators are evaluated first without needing to memorize the entire precedence table can help you write more readable and predictable code.
– Use strict comparison operators (;===> and ;!==>): These ensure that the comparison considers both value and type, which can prevent unexpected results due to type coercion.
– Readable over concise: While it’s tempting to use logical or ternary operators to make your code shorter, prioritize readability, especially when working on projects with others.
Conclusion
Operators in JavaScript are foundational elements that enable you to carry out operations on variables and values. By understanding how to use these symbols effectively, you’ll be able to create more dynamic, efficient, and readable code. Remember, practice is key in mastering these concepts, so don’t hesitate to experiment and apply these operators in different coding scenarios.