Operators in JavaScript: From Basics to Advanced Uses
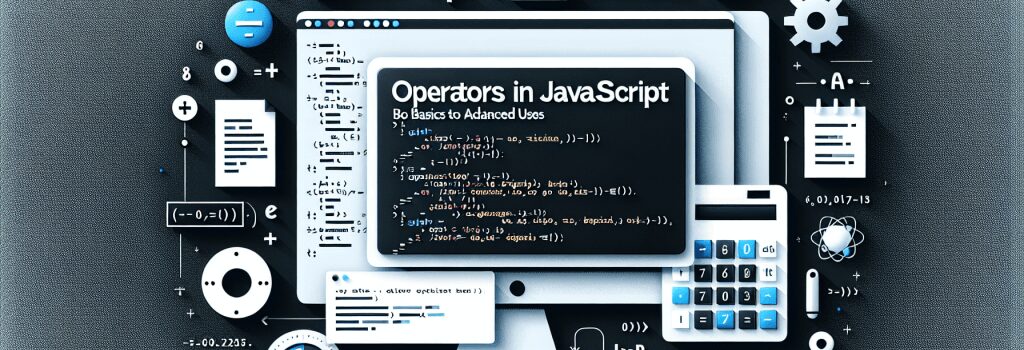
Understanding Operators in JavaScript: The Key to Effective Coding
Introduction to JavaScript Operators
Operators in JavaScript are the building blocks that allow us to perform operations on variables and values. These symbols instruct the browser to carry out mathematical, relational, or logical operations, resulting in dynamic and interactive web experiences. From simple arithmetic to complex logic, understanding operators is essential for any aspiring web developer.
Types of JavaScript Operators
Arithmetic Operators
Arithmetic operators are used to perform common mathematical operations. These include:
– Addition (;+>)
– Subtraction (;->)
– Multiplication (;>)
– Division (;/>)
– Modulus (;%>, which gives the remainder of a division)
– Increment (;++>, adds one to its operand)
– Decrement (;–>, subtracts one from its operand)
These are the bread and butter of mathematical operations in JavaScript and are straightforward to use.
Assignment Operators
Assignment operators are used to assign values to JavaScript variables. The most common assignment operator is the equals sign (;=>), which assigns the value on its right to the variable on its left. There are also compound operators like ;+=>, ;-=>, ;=>, and ;/=>, which combine the assignment with an arithmetic operation.
Comparison Operators
Comparison operators compare two values and return a Boolean value, either true or false. These operators include:
– Equal to (;==>)
– Not equal to (;!=>)
– Strictly equal to (;===> which also checks for same type)
– Strictly not equal to (;!==>)
– Greater than (;>>)
– Less than (;<>)
– Greater than or equal to (;>=>)
– Less than or equal to (;<=>)
Understanding these is crucial for making decisions in your code.
Logical Operators
Logical operators are used to combine or alter Boolean logic. These include:
– Logical AND (;&&>)
– Logical OR (;||>)
– Logical NOT (;!>)
They are commonly used in conditional statements to make more complex conditions.
Advanced Uses of JavaScript Operators
Ternary Operator (Conditional Operator)
The ternary operator is a shortcut for the ;if> statement and is represented as ;condition ? value1 : value2>. If the condition is true, ;value1> is returned; otherwise, ;value2> is returned. It’s a concise way to write simple conditionals.
# Nullish Coalescing Operator (
;??>)Introduced in ES2020, the nullish coalescing operator returns its right-hand side operand when its left-hand side operand is ;null> or ;undefined>, and otherwise returns its left-hand side operand. It is useful in assigning default values.
# Optional Chaining Operator (
;?.>)The optional chaining operator allows developers to safely access deeply nested object properties without having to check if every layer in the chain is valid. It short-circuits and returns ;undefined> if any segment is ;null> or ;undefined>.
Best Practices for Using Operators in JavaScript
1. Choose the Right Operator for the Job: Understanding the subtle differences between operators is key to using them effectively.
2. Clarity Over Conciseness: While shorthand and advanced operators can make your code more concise, always prioritize clear, readable code, especially in complex conditional logic.
3. Debugging: Incorrect use of operators is a common source of bugs. Test your code thoroughly and be aware of the types of values your operators are working with, especially when dealing with strict comparison operators or type coercion.
4. Continuous Learning: JavaScript is always evolving, and so are its operators. Keep up-to-date with the latest features and best practices.
Conclusion
Operators in JavaScript are powerful tools that, when mastered, can greatly enhance your coding skills and allow you to write more concise and efficient code. From basic arithmetic to complex logical expressions, a thorough understanding of how to use these operators is essential for anyone looking to become a proficient web developer. With practice and mindful usage, operators can unlock the full potential of JavaScript in creating dynamic and responsive web applications.