JavaScript Variables in Action: Interactive Elements for Web Pages
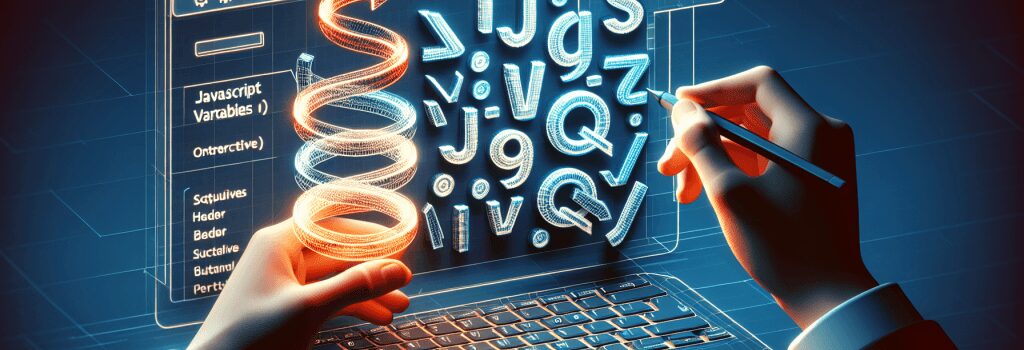
Alright, strap in, eager programmers, because today we are diving into the glorious world of JavaScript variables. Consider variables the spice in your coding soup; without them, things would taste pretty bland. And no one likes bland web pages, do they?
What do we mean by a ‘variable’? In programming language, a variable is a juicy bucket in which we can store values. So, fancy creating a bucket to store your hopes and dreams of becoming a leading software engineer? Awesome! Just kidding. Sadly, JavaScript variables are more about numbers and text than dream collection, but they’re still super cool!
Everything Starts with a Var
Yet don’t rush to name your firstborn ‘Var’, because in JavaScript, ‘var’ actually means ‘variable’. This is where the magic of storing your data begins. When you declare a variable, you’re basically telling JavaScript: “Hey, I need a space to store this piece of data, be it an adorable puppy gif or just a number.”
Here’s how you do it:
;var dreams = “top software engineer”;>
But remember, naming variables is an art. You can’t include spaces, start with numbers, or use some special symbols. Keep it classy, coders.
Let’s Get Dynamic with Data types
Shhh, don’t get scared. It’s not a washed-up 90s boy band. JavaScript gives us a few different data types for our variables:
* Number: Like ;var computers = 4;>
* String: Like ;var country = “Sweden”;>
* Boolean: Like ;var canCode = true;> (We believe in you!)
Operators, Assemble!
Now that we have our variables and data types sorted, let’s give a quick shout-out to operators. No, they’re not the folks wearing headphones in a 1950s telephone exchange. Operators are the symbols that perform operations (blew your mind, huh?) with those super fun variables.
Here are some operators in JavaScript:
* Arithmetic: Like ;+>, ;->, ;*>, ;/>
* Assigning: Like ;=>, ;+=>, ;-=>, etc.
* Comparable: like ;==>, ;===>, ;!=>, ;>>, ;<>
Writing a line like ;dreams += " at Google";> will change your originally declared string to "top software engineer at Google". Operator magic in action!
Creating Interactive Elements on Web Pages
Picture this: You want your website to pop up a little “Hello!” message when somebody visits it. This is where variables turn into superheroes, creating interactive elements! You can create this feature using JavaScript variables and a built-in alert function:
;var greeting = “Hello visitor!”;>
;alert(greeting);>
Voila! Your website is now that friendly neighbour who always says hi.
Now, you’re not just a kid with a dream; you’re a kid with legit coding chops! You’ve put JavaScript variables into action and begun to spice up those web pages. Pat your code-covered back, you’re stepping up in the programming world.
Just remember to keep your variables clean, your operators running, and your data types dynamic — your coding future will thank you. Well, shall we meet in the next chapter where all these skills continue to spiral into a web of wonder?
Keep coding, you future var-veteran. See what we did there? You’re already speaking the language — now that’s progress!