Complex Data Structures in PHP: Understanding Arrays, Objects, and More
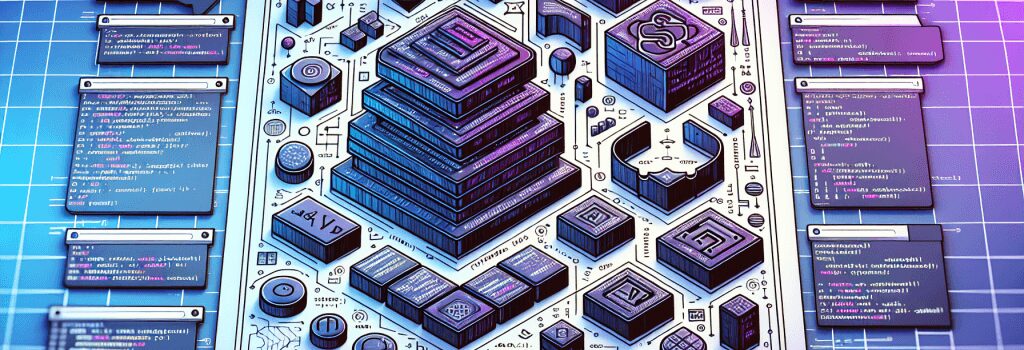
Unlocking the Power of Complex Data Structures in PHP
In the world of web development, understanding complex data structures is pivotal for creating efficient and dynamic websites. PHP, being a server-side scripting language, offers a rich set of data structures, including arrays, objects, and more, to handle data in a web application. This guide aims to demystify these structures, enhancing your development skills and broadening your understanding of PHP.
PHP Arrays Explained
What Are Arrays?
Arrays in PHP are powerful and flexible. They are special variables that can hold more than one value at a time, organized into a collection. Arrays are incredibly useful for storing, managing, and operating on sets of variables.
Types of Arrays in PHP:
1. Indexed Arrays: Store elements with numeric index.2. Associative Arrays: Store elements with named keys.
3. Multidimensional Arrays: Arrays that contain one or more arrays.
How to Use Arrays
Creating an array in PHP is straightforward:For associative arrays:
Arrays offer built-in functions for sorting, merging, slicing, and filtering, making them incredibly versatile for data manipulation.
Diving into PHP Objects
Understanding Objects
Objects in PHP provide a way to group related variables and functions (properties and methods) into a coherent unit. An object is an instance of a class, which can be thought of as a blueprint for the object.
Creating and Using Objects
First, define a class, then instantiate an object of that class:Objects are central to the concept of object-oriented programming (OOP) in PHP, enabling encapsulation, inheritance, and polymorphism.
Beyond Basics: JSON, Serialization, and stdClass
Working with JSON in PHP
JSON (JavaScript Object Notation) is a lightweight data-interchange format. PHP makes it easy to convert arrays and objects to JSON and vice versa, facilitating data exchange between server and client.
Serialization in PHP
Serialization involves converting a PHP object or array into a storable representation. This is useful for storing complex data structures in a database or session.
Using stdClass for Dynamic Properties
stdClass is PHP’s generic empty class, useful for creating objects on the fly without explicitly declaring a class.
Conclusion
Understanding and utilizing complex data structures such as arrays, objects, and more in PHP is crucial for developing robust, efficient, and dynamic web applications. This guide has provided an overview of how these structures work and how you can leverage them in your PHP code. With practice, you’ll find that these concepts are not just theoretical but are practical tools that enhance your coding and problem-solving skills in web development. Embrace these structures, experiment with them, and watch as your proficiency in PHP grows.