Functions in PHP: Creating Reusable Code Blocks
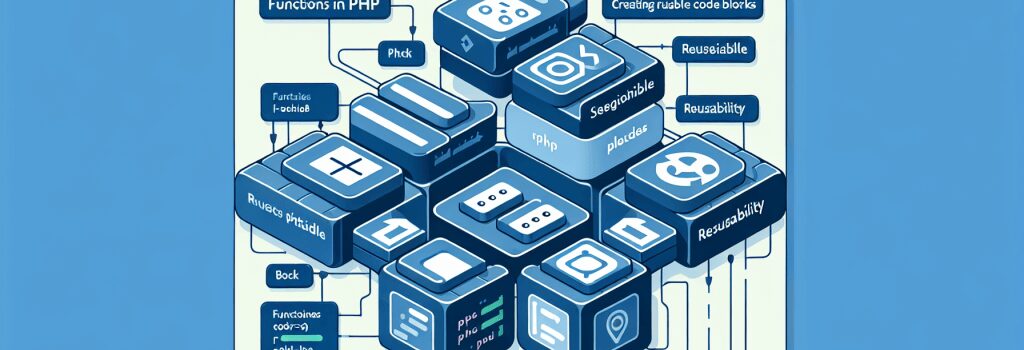
In the realm of backend development, mastering PHP is akin to gaining a key to an extensive library of web development capabilities. Among the many concepts that PHP offers, functions stand out as fundamental building blocks that enable developers to create modular, reusable code. Understanding how to effectively utilize functions in PHP not only streamlines your coding process but also enhances the readability and maintainability of your codebase.
Understanding PHP Functions
PHP functions are encapsulated blocks of code designed to perform a specific task. Once defined, a function can be called multiple times throughout a script, saving time and reducing the likelihood of errors. This concept is crucial in writing efficient code that adheres to the DRY (Don’t Repeat Yourself) principle.
Defining a Function in PHP
Creating a function in PHP begins with the ;function> keyword, followed by a unique function name and a set of parentheses. The function body, enclosed in curly braces ;{}>, contains the code to be executed each time the function is called.
This simple example introduces a function named ;sayHello> that, when called, outputs the string ;Hello, World!>.
Passing Parameters to PHP Functions
Functions become significantly more versatile when they can operate on different data. This is achieved through parameters, which are specified within the parentheses at the time of function definition. Parameters act as placeholders for data that can be passed into the function when it is called.
In this example, the ;greet> function takes one parameter, ;$name>, and uses it to customize the greeting message.
Returning Values from Functions
Beyond performing tasks, functions can also return values using the ;return> statement. Once a return statement is executed, the function will cease execution and output the specified value.
This function, named ;add>, takes two parameters and returns their sum.
Understanding Scope
The scope of a variable defines its accessibility. In PHP, variables declared outside a function are inaccessible within the function unless they are passed as parameters or declared as global.
This snippet demonstrates how to access a global variable within a function using the ;global> keyword.
PHP Built-in Functions
PHP comes with a rich set of built-in functions that can perform a variety of tasks, from manipulating strings and arrays to handling files and dates. Leveraging these pre-defined functions can significantly reduce development time and effort.
Conclusion
Functions in PHP are a powerful feature that enables efficient, modular, and reusable code. By understanding how to define functions, pass parameters, return values, and manage scope, developers can write cleaner, more maintainable PHP scripts. Additionally, taking advantage of PHP’s extensive library of built-in functions can further streamline the development process. As you continue to explore PHP, integrating functions effectively into your projects will undoubtedly be a key factor in your success as a web developer.