Implementing Lazy Loading in JavaScript for Improved Website Performance
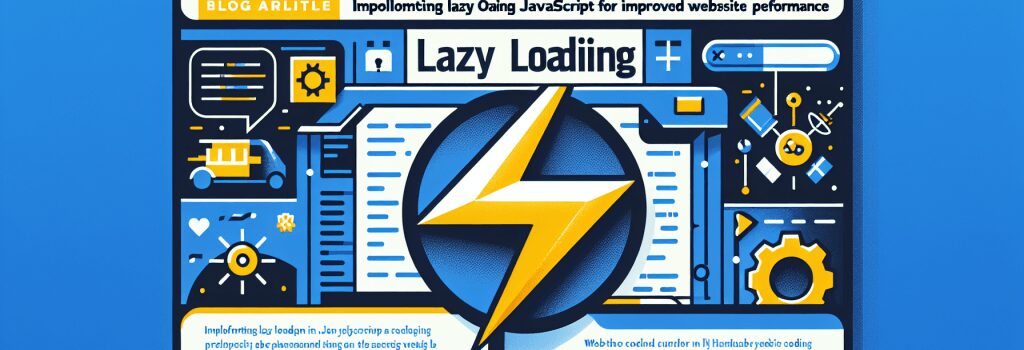
Introduction to Lazy Loading in Web Development
In the modern web development landscape, page load time is a critical factor that impacts user experience and SEO rankings. One effective technique to enhance website performance is implementing lazy loading, particularly for images and scripts. This article will guide you through the process of implementing lazy loading in JavaScript, a strategy designed to significantly improve your website’s loading speed and efficiency.
Understanding Lazy Loading
Before diving into the implementation, it’s essential to understand what lazy loading is. Lazy loading is a design pattern that defers the loading of non-critical resources at page load time. Instead, these resources are loaded at the moment they are needed, which often translates to when they are about to enter the viewport. This method not only speeds up the website’s initial load time but also saves bandwidth by loading less data.
Benefits of Lazy Loading
– Improved Page Load Time: By loading only the essential resources, the initial page load time decreases significantly.
– Reduced Server Load: Lazy loading reduces the number of requests to the server at page load, lightening the server load.
– Enhanced User Experience: Pages become more responsive and accessible, significantly improving the browsing experience.
Implementing Lazy Loading with JavaScript
To implement lazy loading, you will primarily work with the Intersection Observer API, a powerful web API that provides a way to asynchronously observe changes in the intersection of a target element with an ancestor element or with a top-level document’s viewport.
Step 1: Selecting Elements for Lazy Loading
First, you need to identify which elements on your page would benefit from lazy loading. Typically, these are images, iframes, and script tags that are below the fold.
Note: Use the ;data-src> attribute to hold the image URL, which we will later load using JavaScript.
Step 2: Setting Up the Intersection Observer
Create a new Intersection Observer instance. The observer will watch the elements and load them as they come into view.
Step 3: Handling Edge Cases and Browser Compatibility
Be mindful of browser compatibility; not all browsers support the Intersection Observer API natively. For unsupported browsers, consider using a polyfill or an alternative fallback method to implement lazy loading.
Advanced Lazy Loading Strategies
Lazy Loading Background Images
For background images, you can use a similar approach by changing the element’s style property once it is in the viewport.
Lazy Loading Scripts
Scripts that are not critical at page load can also be loaded lazily. This is particularly useful for third-party scripts like analytics or chatbot widgets.
Conclusion
Implementing lazy loading in JavaScript is a powerful optimization technique to improve the performance of your websites. By understanding and applying the principles and steps outlined in this guide, you can achieve faster page loads, reduced server load, and an enhanced user experience. As web development best practices continue to evolve, staying informed and adopting efficient loading strategies will be key to delivering high-quality web applications.
Remember, the ultimate goal of lazy loading and other optimization techniques is to create seamless, engaging experiences for users while maximizing the technical efficiency of your websites. Happy coding!