Posts with tag “JavaScript”
JavaScript is a powerful and essential programming language for web development. In this tag, you will find a collection of posts dedicated to helping you learn and master JavaScript. From the basics of variables and functions to more advanced concepts like DOM manipulation and asynchronous programming, these posts will guide you through everything you need to know to become proficient in JavaScript and enhance your skills as a web developer.
Read more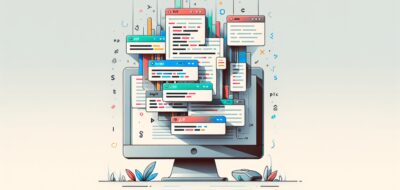
Understanding the Basics of Web Performance Optimization in HTML, PHP, CSS, and JS
---In the dynamic world of web development, ensuring that your website performs at its ...
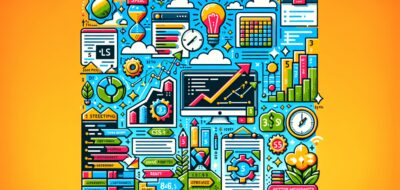
Essential Tips for Optimizing CSS and JavaScript for Better Website Speed
Unlocking Speed: Best Practices for Optimizing CSS and JavaScriptIn the dynamic world of web ...
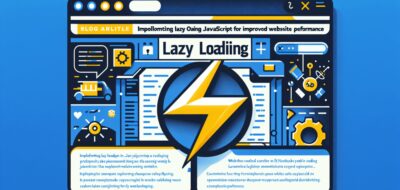
Implementing Lazy Loading in JavaScript for Improved Website Performance
Introduction to Lazy Loading in Web DevelopmentIn the modern web development landscape, page load ...
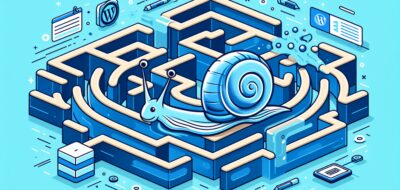
Optimizing WordPress Sites: Best Practices for Speed and Efficiency
In the fast-paced digital world, website speed and efficiency are crucial for maintaining user ...
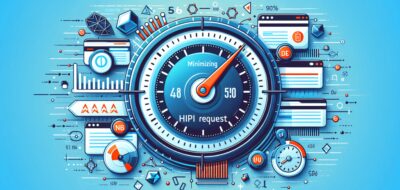
Minimizing HTTP Requests for a Faster Web Experience
Crafting a swift and efficient website is a quintessential goal for web developers aiming ...
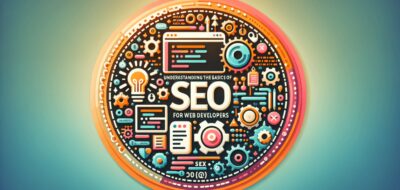
Understanding the Basics of SEO for Web Developers
Understanding the fundamentals of Search Engine Optimization (SEO) is essential for web developers aiming ...
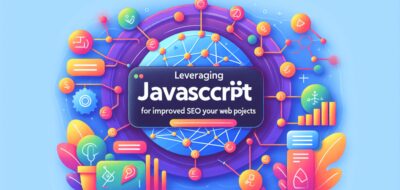
Leveraging JavaScript for Improved SEO on Your Web Projects
In today's digital age, ensuring your web projects are not just visually appealing but ...
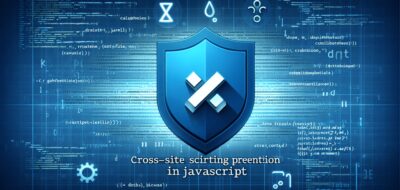
Cross-Site Scripting (XSS) Prevention in JavaScript
Understanding Cross-Site Scripting (XSS) and Its Importance in Web DevelopmentCross-Site Scripting (XSS) is a ...
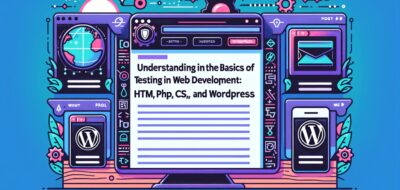
Understanding the Basics of Testing in Web Development: HTML, PHP, CSS, JS, and WordPress
Understanding the Basics of Testing in Web DevelopmentTesting in web development is a critical ...
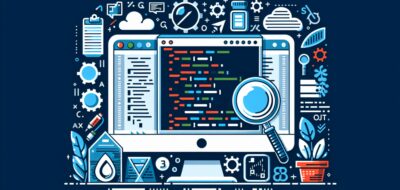
JavaScript Debugging: Tools and Techniques to Enhance Your Web Projects
JavaScript Debugging: Tools and Techniques to Enhance Your Web Projects JavaScript, a cornerstone of ...
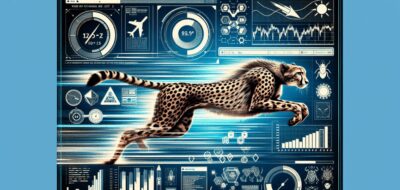
Performance Optimization: Testing and Debugging for Speed
Introduction to Performance Optimization in Web DevelopmentIn the realm of web development, ensuring your ...
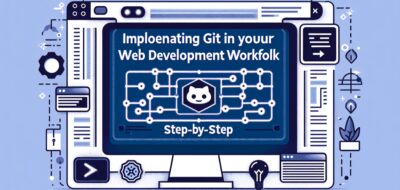
Implementing Git in Your Web Development Workflow: Step-by-Step
Implementing Git into your web development workflow can significantly enhance your coding efficiency, team ...
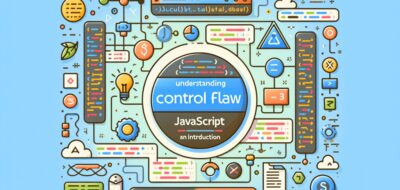
Understanding Control Flow in JavaScript: An Introduction
Understanding how control flow works in JavaScript is crucial for every web developer, from ...
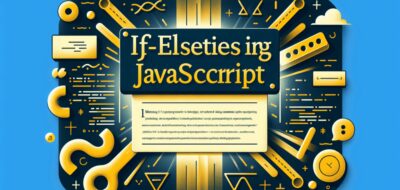
Mastering If-Else Statements in JavaScript
Mastering If-Else Statements in JavaScriptIntroductionIn the world of web development, understanding the basics of ...
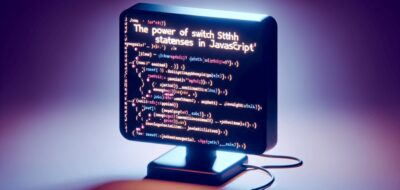
The Power of Switch Statements in JavaScript
Heading: The Power of Switch Statements in JavaScriptIn the realm of web development, mastering ...
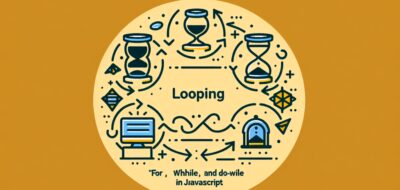
Looping with For, While, and Do-While in JavaScript
Looping structures play a crucial role in JavaScript programming, allowing developers to efficiently execute ...
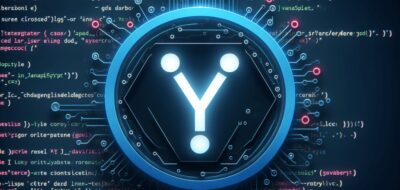
Leveraging Ternary Operators for Concise Code in JavaScript
In the world of JavaScript, efficiency and conciseness are key. With web development continuously ...
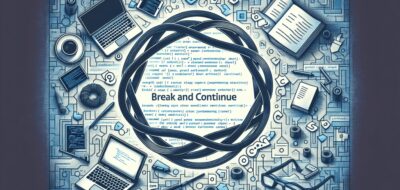
Breaking Down Loop Control Statements: Break and Continue
Understanding Loop Control Statements: Break and Continue in JavaScriptIn the world of web development, ...
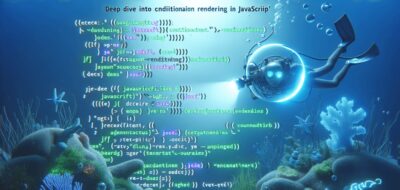
Deep Dive into Conditional Rendering in JavaScript
Introduction to Conditional Rendering in JavaScriptJavaScript powers the dynamic behaviors and interactions on websites, ...
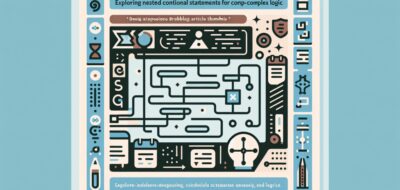
Exploring Nested Conditional Statements for Complex Logic
Understanding Nested Conditional Statements in JavaScriptNested conditional statements are a powerful tool in JavaScript, ...
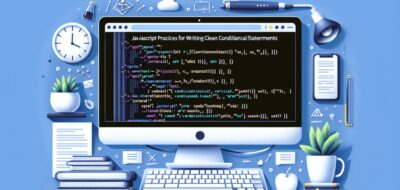
JavaScript Best Practices for Writing Clean Conditional Statements
JavaScript Best Practices for Writing Clean Conditional StatementsWriting clean, efficient, and maintainable code is ...
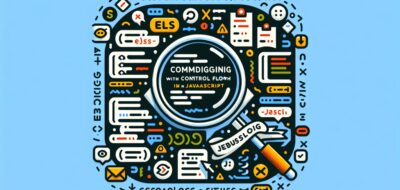
Debugging Common Issues with Control Flow in JavaScript
Introduction to Debugging JavaScript Control FlowWhen embarking on the journey of web development, understanding ...
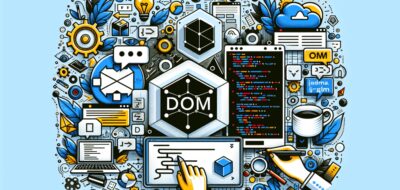
Understanding the Basics of DOM in JavaScript
Introduction to DOM in JavaScriptThe Document Object Model, or DOM, is a crucial concept ...
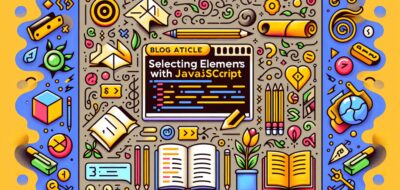
Selecting Elements with JavaScript: A Beginner’s Guide
Selecting elements with JavaScript is a crucial skill for any aspiring web developer. It ...
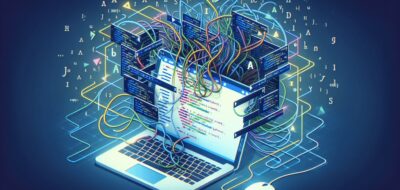
Manipulating HTML Elements Using JavaScript
Manipulating HTML Elements Using JavaScriptIn the modern web development ecosystem, JavaScript stands out as ...
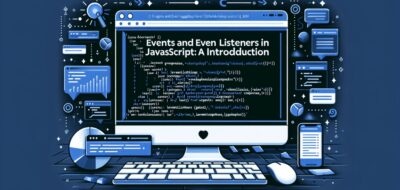
Events and Event Listeners in JavaScript: An Introduction
Events and Event Listeners in JavaScript play a pivotal role in creating interactive web ...
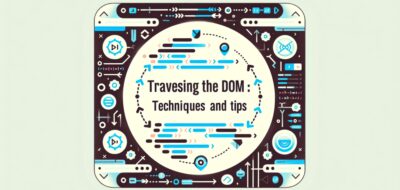
Traversing the DOM: Techniques and Tips
Traversing the DOM: Techniques and Tips The Document Object Model (DOM) is a crucial ...
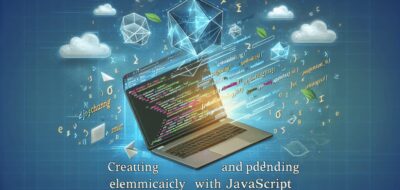
Creating and Appending Elements Dynamically with JavaScript
Understanding Dynamic Element Creation in JavaScriptIn the world of web development, JavaScript stands out ...
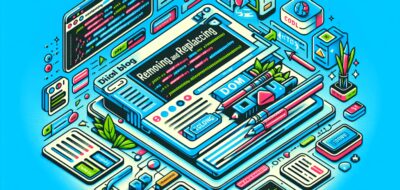
Removing and Replacing Elements in the DOM
---Understanding the DOMBefore delving into the specifics of removing and replacing elements in the ...
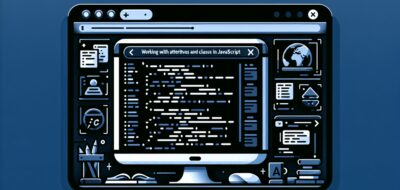
Working with Attributes and Classes in JavaScript
Understanding Attributes and Classes in JavaScriptJavaScript is a powerful programming language that is widely ...