Understanding JavaScript Events and How They Work
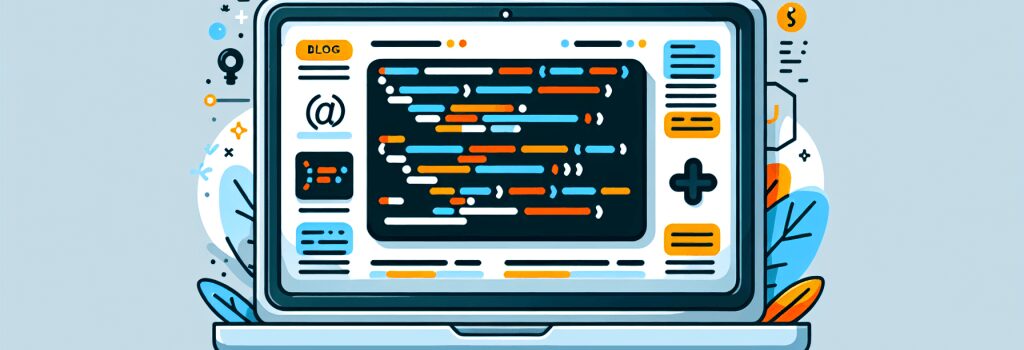
Understanding JavaScript Events and How They Work
JavaScript is a powerful programming language that plays a significant role in making websites interactive and dynamic. One of the core concepts every web developer must grasp is how JavaScript events work. Events are actions or occurrences that happen in the system you’re programming, which the system tells you about so your code can react to them. In web development, these events could be anything from a user clicking a button, submitting a form, moving their mouse, to pressing a key on the keyboard.
What Are JavaScript Events?
JavaScript events are notifications that are sent to notify the code that something has happened on the webpage. These events can be triggered by various actions, including user interactions, browser actions, and even by the web page itself loading or unloading.
User-Generated Events:
– Click: The user clicks on an element.
– Mouseover: The user moves their mouse over an element.
– Keydown: The user presses a key.
Browser-Generated Events:
– Load: The web page has finished loading.
– Resize: The browser window has been resized.
How Events Work in JavaScript
In JavaScript, events follow a flow that enables web developers to interact with their web pages in real-time. This process involves three main steps: event creation, event triggering, and event listening.
Event Creation
Events are created either by user actions or by the browser. For instance, when a user clicks on a button, an event is created. Similarly, when a web page finishes loading, an event is generated.
Event Triggering
Once an event has been created, it is then triggered. This is essentially the event happening. For example, the click event is triggered the moment a user clicks a button.
Event Listening
For an event to have any effect, there must be a listener attached to it. An event listener is a JavaScript function that waits for a specific event to occur and then executes a function in response. Event listeners are attached to elements on the web page and are a crucial part of making pages interactive.
javascript
document.getElementById("myButton").addEventListener("click", function() {
alert("Button clicked!");
});
In the above example, an event listener is added to an element with the ID ;myButton>. When ;myButton> is clicked, the function shows an alert saying "Button clicked!".
Event Propagation: Bubbling and Capturing
Event propagation refers to the order in which events are received on the web page. There are two main types of event propagation: bubbling and capturing.
Bubbling
In bubbling, the event is first captured and handled by the innermost element and then propagated to outer elements. This is the default behavior in JavaScript.
Capturing
Capturing is the opposite process of bubbling. The event starts from the outermost element and progresses to the inner elements.
Developers can choose whether they want to use bubbling or capturing when they attach an event listener by specifying the third parameter in the ;addEventListener> method. Setting it to ;true> uses capturing, while ;false> (or omitting it) uses bubbling.
javascript
// Using capturing
document.getElementById("myButton").addEventListener("click", function() {
alert("Button clicked!");
}, true);
Conclusion
Understanding how events work in JavaScript is fundamental for web developers who aim to create interactive and dynamic web applications. By mastering event handling, you can significantly enhance the user experience on your web pages. Events allow developers to respond to user actions in real-time, making web applications feel more responsive and intuitive. Whether it’s something as simple as a button click or as complex as handling asynchronous data fetching with AJAX, mastering JavaScript events is a crucial step on your journey to becoming an accomplished web developer.