The Role of Data Types in JavaScript Coding
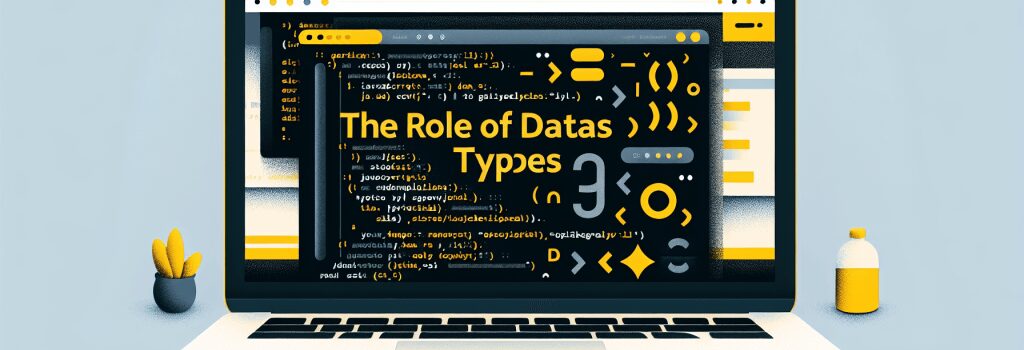
Understanding the Impact of Data Types in JavaScript Programming
In the realm of web development, JavaScript stands as a foundational scripting language, powering interactive features and dynamic content across countless websites. For aspiring web developers delving into JavaScript, comprehending the roles of variables, data types, and operators isn’t just beneficial—it’s essential. This article explores the significance of data types in JavaScript coding, providing insights crucial for developing efficient and error-free code.
The Basics of Data Types in JavaScript
JavaScript is a loosely typed language, meaning variables do not require a declaration of their data type. However, understanding data types is critical as they dictate what operations can be performed on the stored data. JavaScript primarily classifies data into two categories: primitive data types and reference data types.
Primitive Data Types
Primitive data types include:
– ;Number>: Represents both integer and floating-point numbers.
– ;String>: Represents sequences of characters, used for text.
– ;Boolean>: Represents logical values, either ;true> or ;false>.
– ;Undefined>: Represents a variable that has been declared but not assigned a value.
– ;Null>: Represents a deliberate non-value.
– ;Symbol>: Introduced in ES6, used for unique identifiers.
Reference Data Types
Reference data types encompass:
– ;Object>: The base type for most JavaScript objects.
– ;Array>: Used to store multiple values in a single variable.
– ;Function>: Represents code that is to be executed.
Understanding these types is fundamental to handling data correctly and efficiently in your JavaScript projects.
Variables and Data Assignment
Variables in JavaScript are used to store data values. With the introduction of ES6, ;let> and ;const> have been introduced alongside ;var>, providing block-level scoping and enhancing code readability and maintainability. Proper understanding and usage of these declarations will significantly impact the functionality and the reliability of your code.
The Power of Operators
Operators allow us to perform operations on variables and values. JavaScript includes:
– Arithmetic operators (;+>, ;->, ;*>, ;/>, ;%>)
– Assignment operators (;=>, ;+=>, ;-=>, etc.)
– Comparison operators (;==>, ;===>, ;!=>, ;!==>, ;>>, ;=>, ;<=>)
– Logical operators (;&&>, ;||>, ;!>)
Each operator interacts differently with various data types, an understanding of which is pivotal to implementing logic correctly and ensuring your application behaves as expected.
Real-World Applications and Best Practices
In practice, the adept use of data types and operators is instrumental in tasks ranging from simple form validations to complex numerical computations and object manipulations. For example, ensuring that a user input is of a ;String> type, or that a numerical operation isn’t unintentionally performed on a ;String>, is basic yet crucial.
A firm grasp on JavaScript’s data types also aids in debugging, making it easier to spot why your code isn’t working as expected. Paying attention to data types can help prevent common issues such as unintended type coercion, which can lead to challenging bugs.
Tips for Success
– Always declare variables with the appropriate data type in mind.
– Use ;===> and ;!==> for comparisons to avoid unintended type coercion.
– Familiarize yourself with type conversion methods to manage and manipulate data effectively.
– Regularly practice coding challenges that involve different data types and operations.
Conclusion
Mastering data types in JavaScript is a fundamental step toward becoming a proficient web developer. By understanding how different data types work and interact through variables and operators, developers can write more efficient, effective, and error-free code. Embrace the diversity of JavaScript’s data types to unlock the full potential of your web development projects.