Mastering Basic Operators in JavaScript for Effective Coding
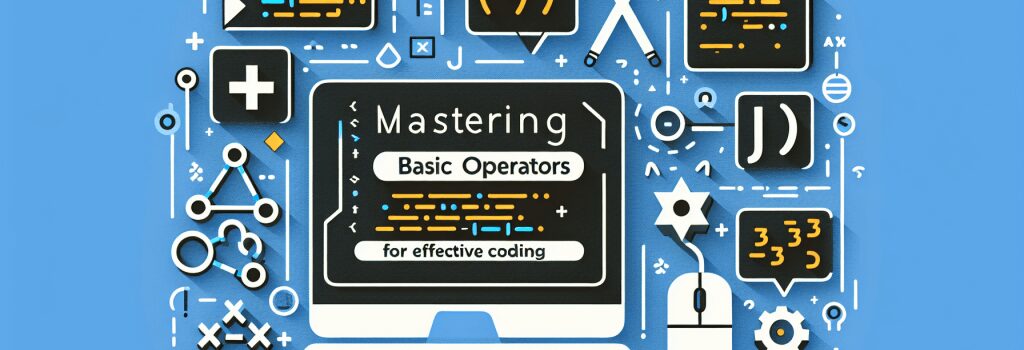
Mastering Basic Operators in JavaScript for Effective Coding
Becoming a proficient web developer requires a firm understanding of JavaScript, the backbone of web interactivity. This article delves into the foundational knowledge of JavaScript, focusing on variables, data types, and, crucially, operators. Operators in JavaScript are symbols that are used to perform operations on operands. They are the building blocks of any JavaScript expression, enabling developers to execute code efficiently and effectively.
Understanding Variables and Data Types in JavaScript
Before diving into operators, it’s essential to grasp the basics of variables and data types. Variables in JavaScript are containers for storing data values. JavaScript supports dynamic typing, meaning you don’t have to declare the type of variable in advance. The data types can be categorized as primitive (undefined, null, boolean, number, string, symbol, bigint) and reference (objects, arrays, functions).
Mastering Basic Operators
JavaScript operators are divided into several categories based on their functionality: arithmetic, comparison, logical, assignment, and others. Each category plays a pivotal role in data manipulation and decision-making processes.
Arithmetic Operators
Arithmetic operators are used for mathematical operations. They include ;+> (addition), ;-> (subtraction), ;> (multiplication), ;/> (division), and ;%> (modulus). Besides these, ;++> (increment), and ;–> (decrement) operators are commonly used to increase or decrease a number by one, respectively.
Assignment Operators
Assignment operators assign values to JavaScript variables. The basic assignment operator is ;=>. However, there are compound operators like ;+=>, ;-=>, ;=>, ;/=>, and ;%=> which combine the arithmetic operations with assignment in one step.
Comparison Operators
Comparison operators compare two values and return a boolean value, true or false, based on whether the comparison is true. These include ;==> (equal to), ;===> (strictly equal to), ;!=> (not equal to), ;!==> (strictly not equal to), ;>> (greater than), ;=> (greater than or equal to), and ;<=> (less than or equal to).
Logical Operators
Logical operators are used to determine the logic between variables or values. The basic logical operators are ;&&> (logical AND), ;||> (logical OR), and ;!> (logical NOT). These operators are pivotal in making decisions based on multiple conditions.
Understanding Operator Precedence
Operator precedence in JavaScript determines how operators are parsed concerning each other. Operators with higher precedence become operands of operators with lower precedence. Understanding the precedence rule is crucial for writing clear and efficient code.
Best Practices for Using Operators in JavaScript
– Understand the difference between ;==> and ;===>. Always use the strict comparison operators (;===> and ;!==>) unless there is a specific reason not to, as they avoid type coercion.
– Utilize shorthand operators for cleaner and more concise code.
– Keep operator precedence in mind to avoid unintended results without the need for unnecessary parentheses.
– Test logical expressions thoroughly, especially if they involve multiple conditions and logical operators.
Mastering the use of basic operators in JavaScript is a fundamental step towards becoming an effective coder. By understanding how to manipulate variables, perform operations, and evaluate conditions, you’re well on your way to developing dynamic web applications. Remember, practice is key—experiment with different operators and scenarios to solidify your understanding and skills.