Leveraging Variables, Data Types, and Operators in JavaScript for Web Development
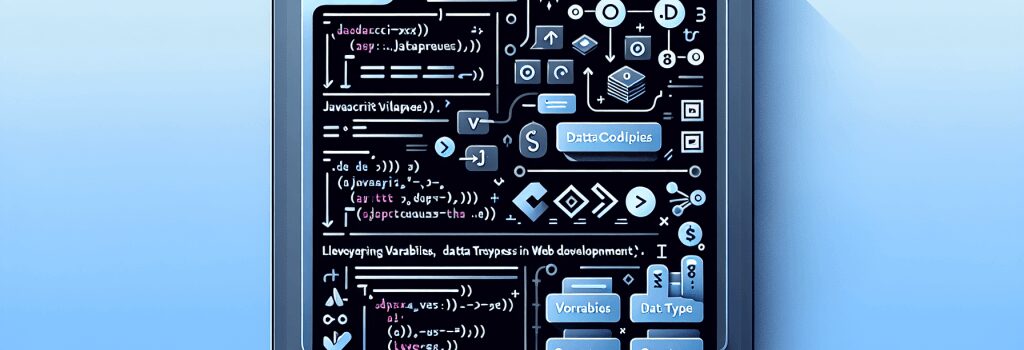
Understanding the Building Blocks of JavaScript
JavaScript stands as a cornerstone of web development, powering dynamic and interactive elements on websites across the globe. As a novice or seasoned web developer, mastering the essentials of JavaScript, including variables, data types, and operators, is crucial for constructing robust and efficient web applications. This article delves into these foundational elements, providing insights on how to leverage them effectively in your web development projects.
Variables: The Essence of Data Handling in JavaScript
Variables: Storing and Managing Data
At its core, a variable in JavaScript is a symbolic name for a value. Variables are containers that hold data which can be manipulated and retrieved throughout the code. Understanding variables is the first step towards managing data within your web applications effectively.
Declaring Variables
JavaScript offers three keywords for declaring variables: ;var>, ;let>, and ;const>. Each serves a different purpose:
– ;var> is used in older JavaScript code. It has a function scope or global scope if not declared inside a function.
– ;let> provides block scope, making it a preferred choice for variables that change, such as counters in loops.
– ;const> is similar to ;let> in terms of scope but is used for variables that should not be reassigned after their initial declaration.
Data Types: Understanding What Your Data Represents
Grasping JavaScript Data Types
JavaScript categorizes data into several types, enabling developers to perform various operations and manipulations. The main types are Number, String, Boolean, Undefined, Null, Symbol (introduced in ES2015), BigInt (a more recent addition for handling large integers), and Object, which includes arrays and functions.
Primitive vs. Reference Types
Understanding the difference between primitive (immutable) and reference (mutable) types can significantly improve your data manipulation strategies. Primitive types are stored by value, while reference types are stored by reference, making them more complex but also more versatile in their application.
Operators: Performing Operations on Your Data
Leveraging JavaScript Operators
Operators in JavaScript are symbols that perform operations on variables and values. These include arithmetic operators (;+>, ;->, ;*>, ;/>, ;%>), comparison operators (;==>, ;!=>, ;===>, ;!==>, ;>), logical operators (;&&>, ;||>, ;!>), and more. Understanding how to use these operators is crucial for implementing logic and algorithms in your code.
Conditional (Ternary) Operator
One powerful yet underutilized operator is the conditional (ternary) operator (;condition ? valueIfTrue : valueIfFalse>). It provides a concise way to execute simple conditional statements, making your code cleaner and more readable.
Incorporating Variables, Data Types, and Operators in Web Development
Understanding and utilizing variables, data types, and operators are fundamental in any web development project. They form the backbone of JavaScript programming, enabling developers to handle data effectively, implement logic, and manipulate web page elements dynamically.
As you progress in your web development journey, continually exploring these concepts will allow you to create more efficient, robust, and interactive web applications. Remember, practice is key. Experiment with different data types, variables, and operators, and observe how they interact within your code. This hands-on experience is invaluable, offering deeper insights into the power of JavaScript in web development.
By strengthening your foundation in these areas, you’re not just learning to code; you’re learning to think like a developer. This mindset, coupled with a solid understanding of JavaScript’s core concepts, will equip you to tackle complex web development challenges, paving the way for your success in the dynamic world of web development.