Exploring the Document Object Model (DOM) with JavaScript
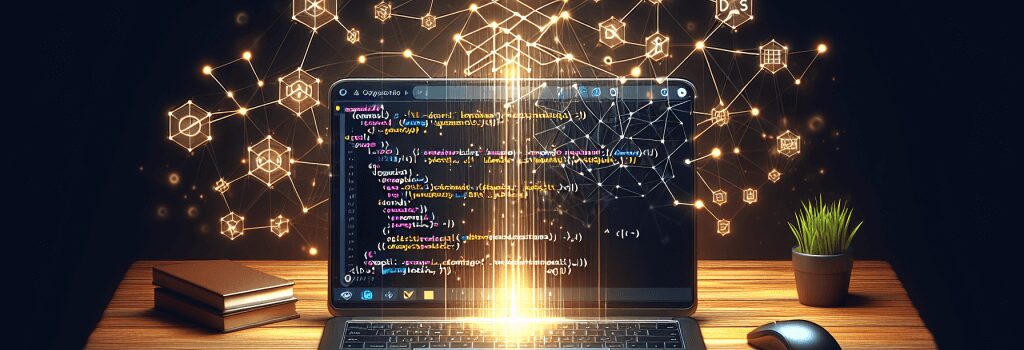
Understanding the Document Object Model (DOM) in JavaScript
When embarking on your journey to become a skilled web developer, one of your essential learning milestones involves mastering the Document Object Model (DOM) with JavaScript. The DOM plays a pivotal role in web development, allowing developers to create, modify, and interact with HTML and XML documents programmatically. This article aims to demystify the DOM, making it an approachable concept for beginners and reinforcing its importance in the realm of web development.
What is the Document Object Model (DOM)?
The Document Object Model (DOM) is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content. The DOM represents the document as a tree of objects; each branch of the tree ends in a node, and each node contains objects. In simpler terms, the DOM is the way JavaScript sees its containing pages’ contents as an object that can be modified with a scripting language like JavaScript.How JavaScript Interacts with the DOM
JavaScript interacts with the DOM through various methods and properties provided by the DOM API (Application Programming Interface). These interactions allow developers to:– Modify an element’s content or style: By accessing and altering the DOM, JavaScript can change the text inside an HTML element or its CSS styling.
– Add or remove elements: JavaScript can dynamically add new elements to the page or remove existing ones, allowing for a more interactive user experience.
– Respond to user actions: By listening for and responding to user events like clicks, keyboard presses, or mouse movements, JavaScript can execute code in response, making web pages responsive and interactive.
Exploring DOM Elements
Understanding DOM elements is fundamental in mastering JavaScript’s manipulation of web pages. Here’s a breakdown of how to work with these elements:– Accessing elements: You can access elements in the DOM using methods like ;getElementById()>, ;getElementsByClassName()>, ;getElementsByTagName()>, or more modern methods like ;querySelector()> and ;querySelectorAll()>.
– Changing elements: Once you’ve accessed an element, you can change its properties, attributes, and styles. For example, you can modify an element’s inner HTML or style it differently using JavaScript code.
– Event handling: Events are crucial for creating interactive pages. JavaScript allows you to add event listeners to elements. When the specified event occurs, your code responds accordingly, enhancing the user’s experience on the page.
Best Practices for DOM Manipulation
While the power to change web pages dynamically offers infinite possibilities, it’s important to follow best practices for efficient and effective DOM manipulation:1. Minimize direct DOM interactions: Each DOM manipulation can affect the page’s performance. Where possible, minimize direct DOM interactions, or update elements off-screen before bringing them to the display.
2. Use efficient selectors: Opt for the most efficient method when selecting elements to manipulate. For example, ;getElementById> is faster than ;querySelector> if you’re selecting by ID.
3. Batch your changes: When making multiple changes to the DOM, consider batching these changes to minimize reflow and repaint processes, enhancing your website’s performance.
In Conclusion
Mastering the Document Object Model (DOM) with JavaScript is a cornerstone of web development, essential for creating dynamic, interactive websites. By understanding and applying the concepts and practices outlined in this article, you’ll be well on your way to leveraging the full potential of JavaScript in manipulating web pages. Remember, practice is key to becoming proficient with the DOM, so experiment with these concepts and continue building your web development skills.FAQ
What is the Document Object Model (DOM) in web development?
The DOM is a programming interface for web documents that represents the structure of the HTML, XHTML, or XML document as a tree where each node in the tree corresponds to a part of the document.
How does JavaScript interact with the DOM?
JavaScript can manipulate the DOM by selecting elements, changing their content, attributes, or styles, and responding to user interactions like clicks or key presses.
What are some common methods to access DOM elements in JavaScript?
Common methods to access DOM elements include getElementById, getElementsByClassName, querySelector, querySelectorAll, and more.
How can events be handled in the DOM with JavaScript?
Events such as click, keypress, mouseover, etc., can be handled using event listeners in JavaScript to trigger specific actions in response to user interactions.
What is event propagation and how does it work in the DOM?
Event propagation refers to the way events flow through the DOM hierarchy from the target element to its ancestors or descendants. It follows either the capturing phase, the target phase, or the bubbling phase.
What is the difference between innerHTML and textContent in the DOM?
innerHTML sets or returns the HTML content inside an element, while textContent sets or returns the text content of the element, excluding any HTML tags.
How can you create new DOM elements dynamically with JavaScript?
New DOM elements can be created dynamically using methods like createElement and appendChild to add elements to the document structure on-the-fly.
How can you remove elements from the DOM using JavaScript?
You can remove elements from the DOM by targeting the parent element and using methods like removeChild or remove to delete specific nodes.
What is the concept of event delegation in DOM manipulation?
Event delegation involves attaching a single event listener to a parent element to manage events on its children, reducing the number of event handlers and improving performance.
How does JavaScript aid in making dynamic and interactive web pages using the DOM?
JavaScript allows for dynamic manipulation of content, styling, and behavior of web pages by interacting with the DOM, providing a seamless user experience and responsive web applications.