Developing Secure Web Forms with HTML and PHP
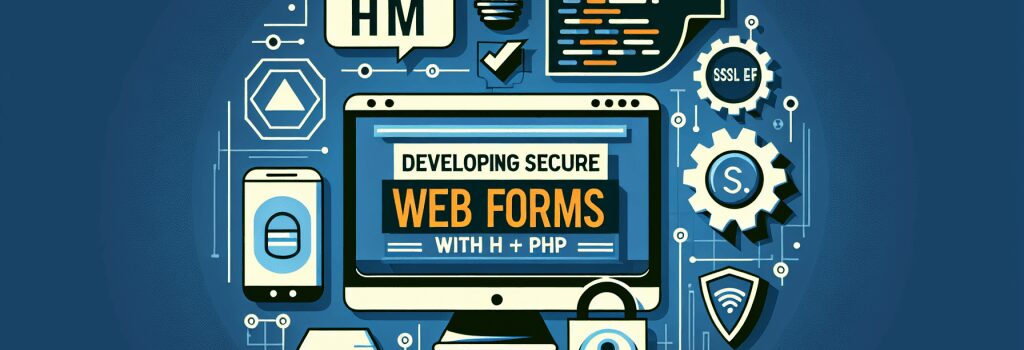
Developing Secure Web Forms with HTML and PHP
In the modern web development landscape, security is paramount, especially when it comes to handling user input through web forms. HTML and PHP offer robust tools to design and secure web forms against common vulnerabilities. This article delves into best practices for creating secure web forms utilizing HTML for structure and PHP for backend processing.
Understanding Web Form Security
Before diving into the technicalities, it’s important to understand why web form security is critical. Web forms are a primary vector for various attacks, including SQL injection, Cross-Site Scripting (XSS), and Cross-Site Request Forgery (CSRF). Protecting against these threats is essential to safeguard user data and maintain trust.
Securely Structuring Forms with HTML
Form Method and Action Attributes
When creating forms with HTML, always specify the method and action attributes securely. Use ;POST> method for forms that submit sensitive data, as it doesn’t append data to the URL, unlike ;GET>.
Validation with HTML5
HTML5 introduces form validation attributes like ;required>, ;type="email">, and ;pattern>, which provide an initial layer of client-side validation:
Processing Forms Securely with PHP
After structuring your forms securely with HTML, the next step involves processing the form data securely with PHP.
Data Sanitization and Validation
Always validate and sanitize user input on the server side to prevent injection attacks. PHP offers functions like ;filter_input()> and ;filter_var()> for sanitizing user data:
Prepared Statements for Database Interaction
If your form data interacts with a database, use prepared statements with PDO (PHP Data Objects) or MySQLi to prevent SQL injection attacks. Prepared statements ensure that user input is treated as data, not as executable code.
Implementing CSRF Protection
A CSRF attack can trick a browser into submitting a forged request to your site. Mitigate this risk by including a unique token in your forms and validating it upon submission.
Using HTTPS
Secure your forms by serving your website over HTTPS. This encrypts the data transmitted between the browser and your server, protecting it from interceptors. Implementing HTTPS is straightforward with the help of Let’s Encrypt, a free, automated, and open Certificate Authority.
Conclusion
Creating secure web forms requires a comprehensive approach that spans both client-side and server-side practices. Leveraging HTML for form structure and PHP for processing, while adhering to security best practices, can significantly mitigate risks associated with web forms. Remember to validate and sanitize all user input, use prepared statements for database interactions, protect against CSRF attacks, and serve your forms over HTTPS to ensure the security of user data. With these strategies in place, you can create safe and reliable web forms for your users.