Understanding PHP Control Structures: If, Else, and Switch
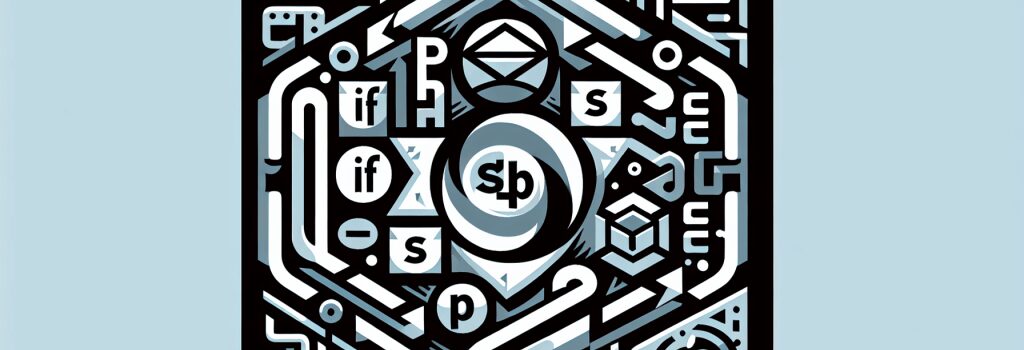
In the journey of mastering backend development with PHP, a fundamental step is to comprehend the workings of control structures. PHP, a server-side scripting language, is equipped with several control structures, but the focus here will be on three primary ones: ;if>, ;else>, and ;switch>. Understanding these structures enhances your ability to make decisions in your code, allowing for more dynamic and interactive web applications.
PHP If Statement
The ;if> statement is the most basic of all control structures in PHP. It executes a block of code if a specified condition is true. The basic syntax of an ;if> statement is as follows:
php
if (condition) {
// Code to execute if condition is true
}
This structure allows your script to perform different actions based on different inputs or conditions. For example, you might use an ;if> statement to check if a user’s input matches a certain criterion.
Understanding If Else
Building on the ;if> statement, the ‘else’ clause extends functionality by executing an alternative block of code if the original condition is false. The syntax is as follows:
php
if (condition) {
// Code to execute if condition is true
} else {
// Code to execute if condition is false
}
This dual-path approach allows for more nuanced decision-making in your PHP applications, directly impacting the user experience based on their interactions or inputs.
Mastering Elseif
The ;elseif> statement is a further extension that allows you to evaluate multiple conditions sequentially. Once a condition is met, its associated code block is executed, and the structure is exited. The syntax:
php
if (condition1) {
// Code to execute if condition1 is true
} elseif (condition2) {
// Code to execute if condition2 is true
} else {
// Code to execute if neither condition1 nor condition2 is true
}
This construct is particularly useful for creating multiple pathways in your applications, handling various scenarios with efficient code.
Diving Into the Switch Statement
The ;switch> statement provides an alternative to multiple ;if> statements when dealing with conditions that involve the same variable or expression. It compares the value of an expression against multiple case values and executes the block of code associated with the first matching case. The syntax is as follows:
php
switch (n) {
case label1:
// Code to execute if n=label1
break;
case label2:
// Code to execute if n=label2
break;
default:
// Code to execute if n is different from all labels
}
The ;switch> statement streamlines decision-making processes in your code, making it cleaner and more readable, especially when you have a large number of conditions to check.
Best Practices with Control Structures
When using control structures in PHP, keeping your code clean and readable is essential. Some best practices include:
– Use consistent indentation to make your code structure clear.
– Employ meaningful variable names that reflect their purpose in conditions.
– Always use ;break> in each ;case> of a switch to prevent unintentional fall-through.
– In ;if> statements, place the most likely condition to be true first to optimize performance.
By mastering PHP control structures, including ;if>, ;else>, and ;switch>, you elevate your backend development skills, enabling the creation of dynamic, efficient, and responsive web applications. These fundamental elements of PHP are pivotal for any aspiring web developer looking to make an impact in the world of web development.