Manipulating PHP Strings for Dynamic Web Content
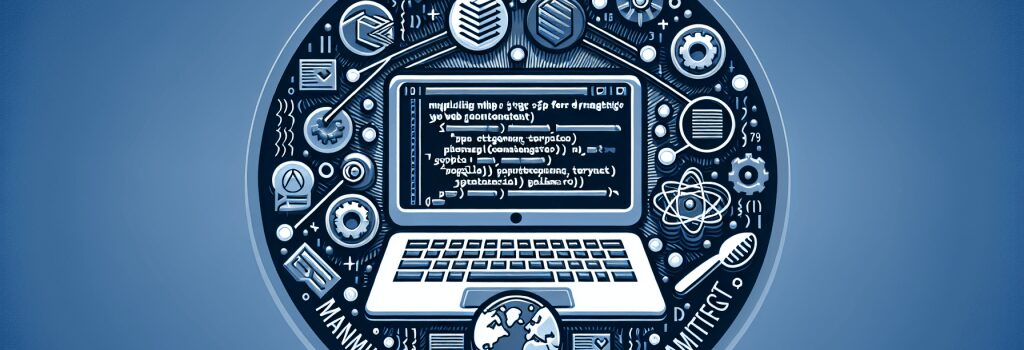
Creating dynamic web content is an essential skill for web developers aiming to deliver a personalized and interactive user experience. PHP, as a server-side scripting language, provides a robust set of features for string manipulation, enabling developers to generate dynamic web content efficiently. In this guide, we’ll explore various PHP string functions that can significantly enhance your backend development skills.
Understanding PHP Strings
Before diving into string manipulation, it’s essential to understand what strings are in the context of PHP. A string is a sequence of characters, like words or sentences, that can be manipulated to dynamically generate content on a webpage. PHP treats strings as an array of bytes, offering an extensive range of functions for string operations.
Common PHP String Functions
PHP provides numerous built-in functions for string manipulation, making it easier for developers to handle and modify strings according to their needs. Here are some of the most useful string functions in PHP:
Working with String Length
– ;strlen()>: The ;strlen()> function calculates the length of a string, which is useful for validation, loops, and conditionals.
Manipulating Case
– ;strtoupper()> and ;strtolower()>: These functions convert a string to uppercase and lowercase, respectively, often used for standardizing data input.
Searching and Replacing
– ;strpos()>: Finds the position of the first occurrence of a substring in a string, useful for search functionalities.
– ;str_replace()>: Replaces all occurrences of a search string with a replacement string in a given string, perfect for dynamic content generation.
Formatting Strings for Dynamic Content
– ;sprintf()>: Allows you to format strings with placeholders, making it incredibly efficient for templating.
Best Practices for String Manipulation
– Validate input data: Always validate and sanitize user inputs to avoid security vulnerabilities, such as SQL injection or cross-site scripting (XSS).
– Use multibyte string functions for UTF-8: When dealing with multilingual content or Unicode characters, use the ;mb_*> functions (e.g., ;mb_strlen>, ;mb_strtolower>) to ensure accurate handling of UTF-8 strings.
– Optimize for performance: Use built-in PHP string functions instead of writing custom functions for common tasks, as they are optimized for performance.
Conclusion
PHP’s powerful string manipulation capabilities are indispensable for developing dynamic web content. By mastering these functions, you can create personalized, interactive websites that engage users effectively. Experiment with these functions and best practices in your projects to harness the full potential of PHP for backend development. Remember, the key to becoming proficient in web development is continuous learning and practice.