Advanced PHP: Anonymous Functions and Closures
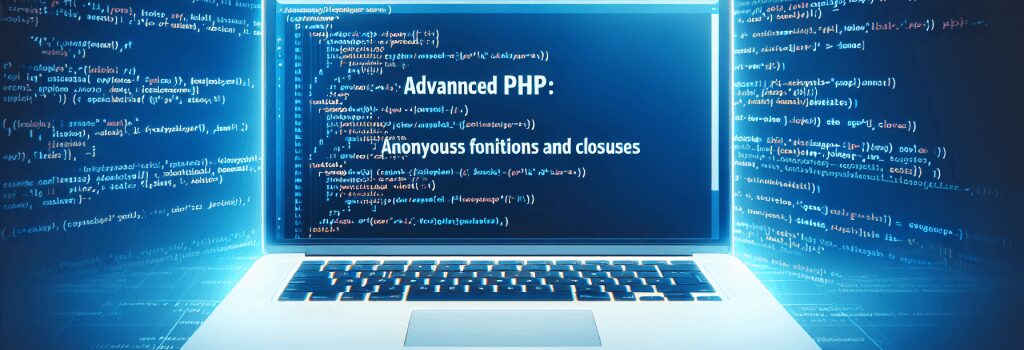
Advanced Techniques in PHP: Embracing Anonymous Functions and Closures
In the realm of backend development, PHP stands out for its ease of use and dynamic capabilities. As you progress from beginner to advanced PHP programming, understanding the power of anonymous functions and closures becomes imperative. These features offer flexibility and elegance in code design, allowing for more readable, maintainable, and concise coding practices.
Understanding Anonymous Functions in PHP
Anonymous functions, also known as closures or lambda functions, are functions without a specified name. They can be stored in variables, passed as arguments to other functions, or even returned from functions. This feature of PHP enables developers to create highly dynamic and flexible code.
Creating Anonymous Functions
Creating an anonymous function in PHP is straightforward. It follows the standard function definition in PHP, except that the function name is omitted. Here’s a basic example:
In this example, the anonymous function is assigned to the variable ;$greet>, which can then be called like any other function:
Leveraging Closures for Enhanced Functionality
Closures are a special kind of anonymous function that have access to variables from the outer scope. This feature enables the function to maintain state between calls without relying on global variables or static function variables.
Closures and Scope
One of the unique aspects of closures in PHP is their ability to ‘inherit’ variables from the parent scope. This is achieved using the ;use> keyword:
This capability introduces a level of flexibility and expressiveness to PHP functions that were previously difficult or messy to implement.
Practical Uses of Anonymous Functions and Closures
Anonymous functions and closures are not merely academic concepts but have practical applications in real-world programming:
– Event Handlers and Callbacks: They can be used as callbacks for event handlers or as arguments for functions expecting a callable, making your code more concise and readable.
– Array Operations: PHP’s array functions such as ;array_map()>, ;array_filter()>, and ;array_reduce()> can leverage anonymous functions to manipulate arrays in a powerful and expressive manner.
– Encapsulating Logic: Closures can encapsulate specific logic or state within a function, reducing the need for global variables or complex class structures for simple tasks.
Best Practices
While anonymous functions and closures offer significant benefits, it’s essential to use them judaniciously to maintain code readability and performance:
– Resource Management: Anonymous functions can increase memory consumption if not used carefully, especially in loops or high-volume operations.
– Readability: Overusing closures can make your code harder to follow. Use them when they bring clear benefits to readability and maintainability.
In conclusion, mastering anonymous functions and closures is a significant step towards advanced PHP development. They not only provide a powerful tool for creating expressive and flexible code but also encourage a deeper understanding of function scope and state management. Embrace these concepts, and you’ll find yourself writing more efficient, readable, and elegant PHP code.