PHP Puzzles: Solve These to Become a Backend Pro
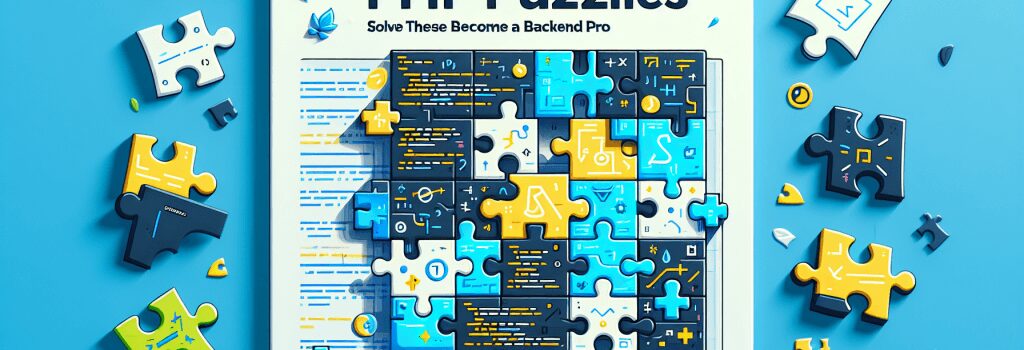
PHP is a pivotal language in the world of web development, powering vast portions of the internet with its robust features and flexibility. Mastering PHP is essential for any aspiring backend developer, and one of the most effective and enjoyable ways to hone your skills is through tackling PHP puzzles and challenges. This article dives into why solving PHP puzzles can elevate your backend prowess and where you can find resources to test and enhance your PHP skills, even without external links. Get ready to boost your coding capabilities and edge closer to becoming a backend development pro.
The Benefits of PHP Puzzles
Sharpen Problem-Solving Skills
Becoming proficient in PHP isn’t just about memorizing syntax; it’s about learning to think like a developer. PHP puzzles force you to confront real-world problems, requiring creative and efficient solutions. By facing various challenges, you learn to navigate through errors and unexpected outcomes, sharpening your problem-solving skills in the process.
Understand PHP In-Depth
PHP puzzles often touch on lesser-known features or quirks of the language. Tackling these puzzles can deepen your understanding of the language, beyond just the basics. It’s one thing to know the syntax, but understanding how and why PHP operates the way it does under different circumstances can truly elevate your programming skills.
Improve Debugging Skills
Many PHP puzzles are designed not just to test your coding ability but also to improve your debugging skills. Debugging is an invaluable skill in a developer’s toolset, significantly impacting development speed and efficiency.
Get Prepared for Job Interviews
Many tech companies include coding challenges as part of their interview process. Being adept at solving puzzles can give you a significant advantage. These challenges are often similar to the types of problems you’ll encounter in PHP puzzles, making regular practice an excellent way to prepare.
Where to Practice: Coding Challenges and Practice Websites
While this guide won’t directly link to resources, it’s worth mentioning a general approach to finding quality PHP puzzles and challenges.
Search For PHP Challenges
A simple search for “PHP coding challenges” or “PHP puzzles” in your preferred search engine will yield a variety of resources. Look for websites that offer a range of difficulties, ensuring that you can start at a level that’s comfortable for you and progress as your skills improve.
PHP Forums and Communities
PHP has a vibrant community of developers who create and share challenges. Forums and PHP-focused groups can be an invaluable resource for finding puzzles that range from beginner to advanced levels.
Coding Practice Websites
While some websites are language-agnostic, they still offer a considerable amount of content relevant to PHP. These platforms often include interactive elements, allowing you to write and test your code within the browser.
Project-Based Learning
Beyond individual puzzles, consider embarking on small projects. While not a “puzzle” in the traditional sense, projects can present numerous mini-challenges that require you to apply your PHP knowledge in practical, often complex scenarios.
Conclusion
Solving PHP puzzles is more than just a way to pass the time. It’s a method of actively engaging with the language, understanding its nuances, and improving your problem-solving abilities. The skills you develop by tackling these challenges are directly applicable to real-world development tasks, making you a more capable and versatile backend developer.
Remember, becoming proficient in PHP—or any programming language—is a journey. Each puzzle solved is a step forward in that journey. So, embrace the challenges, learn from your mistakes, and continue pushing your boundaries. Happy coding!