Data Types and Variables in JavaScript: A Comparative Study
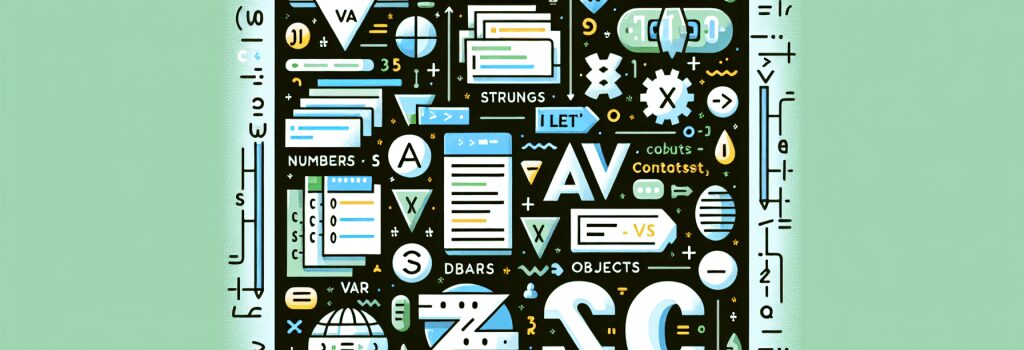
Introduction to Data Types and Variables in JavaScript
When embarking on the journey of becoming a web developer, one of the foundational steps is to grasp the concepts of data types and variables in JavaScript. As a key component of the JavaScript foundations, understanding these concepts is critical for programming not just for the web, but for any environment where JavaScript plays a role. This article aims to illuminate the differences and uses of various data types and variables in JavaScript, laying a robust foundation for budding developers.
Understanding Variables in JavaScript
Variables in JavaScript can be thought of as containers for storing data values. In JavaScript, there are three main ways to declare a variable: using the keywords ;var>, ;let>, or ;const>.
– var: Declares a variable, optionally initializing it to a value.
– let: Declares a block-scoped, local variable, optionally initializing it to a value.
– const: Declares a block-scoped, read-only named constant.
Var vs. Let vs. Const
– The ;var> keyword declares a function-scoped or globally-scoped variable, and can be re-declared and updated.
– The ;let> keyword declares a block-scoped local variable, and can be updated but not re-declared.
– The ;const> keyword declares a block-scoped variable that cannot be re-declared or updated.
Data Types in JavaScript
JavaScript is a loosely typed or a dynamic language, meaning you don’t need to declare a variable’s type ahead of time. The types of data can be broadly categorized into two: primitive data types and reference data types.
Primitive Data Types:
1. String: Represents a sequence of characters used to represent text.
2. Number: Represents both integer and floating-point numbers.
3. BigInt: Can represent integers with arbitrary precision.
4. Boolean: Represents a logical entity and can have two values: true and false.
5. Undefined: Represents a variable that has not been assigned a value.
6. Null: Represents the intentional absence of any object value.
7. Symbol: Represents a unique, immutable primitive value and can be used as the key of an Object property.
Reference Data Types:
– Object: The most important data-type and forms the building blocks for modern JavaScript. Objects can be created with braces ;{}> with an optional list of properties.
– Array: A global object that is used in the construction of arrays; which are high-level, list-like objects.
Comparative Study Between Primitive and Reference Data Types
The main difference between primitive and reference data types lies in how they are stored and accessed in memory. Primitive types store their values directly in the location the variable accesses, while reference types store a pointer to the location in memory where the data is stored. This distinction is crucial as it affects how you work with the values in your code, especially when it comes to mutation and comparison of variables.
Operational Behaviors
– Copying Variables: Copying a primitive type from one variable to another copies the value directly. For reference types, however, it is the memory address that is copied, not the actual value.
– Comparison of Variables: Comparing primitive types is straightforward and is done by value. For reference types, comparison is done by the reference (memory address), not by the actual value.
Conclusion
Understanding the nuances of data types and variables in JavaScript is foundational for any web developer. It influences how you structure your data, how you write your code, and how efficient and maintainable that code is. By distinguishing between the primitive and reference data types, and understanding the use of ;var>, ;let>, and ;const> for declaring variables, developers can write cleaner, more effective code that leverages the rich capabilities of JavaScript to build engaging, dynamic web experiences. As you continue exploring JavaScript, let this knowledge serve as the bedrock upon which you construct increasingly complex and powerful web applications.