Understanding Variables in JavaScript: A Beginner’s Guide
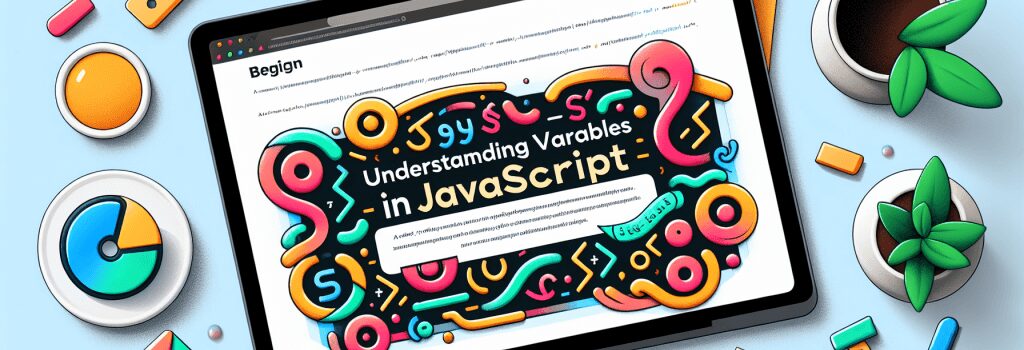
Learning the basics of JavaScript is pivotal for any aspiring web developer. Among the foundational concepts, understanding variables is paramount. Variables act as containers for storing data values. In JavaScript, they play a significant role in data manipulation, making your web applications interactive and dynamic. This guide breaks down what variables are, how to use them, the various data types you’ll encounter, and the operators used to manipulate data, offering a comprehensive beginner’s overview.
What are Variables in JavaScript?
In JavaScript, variables are used to store data values. Think of them as named boxes you can use to hold pieces of information. Once a variable is declared, you can refer back to it and the data it holds throughout your code. JavaScript uses the ;var>, ;let>, and ;const> keywords for variable declarations, each with its own scope and usage rules.
Declaring Variables
To declare a variable in JavaScript, you can use the ;var>, ;let>, or ;const> keyword followed by a name (identifier) for your variable:
javascript
var name = 'John';
let age = 30;
const isDeveloper = true;
– ;var> declares a variable with function scope or globally if declared outside a function.
– ;let> allows you to declare variables with block scope.
– ;const> is used to declare constants with block scope, meaning the variable cannot be reassigned.
Naming Variables
Variable names in JavaScript must begin with a letter, underscore (_), or dollar sign ($). They can contain letters, digits, underscores, and dollar signs. Variable names are case-sensitive and should be descriptive of the data they hold.
Understanding Data Types and Values
JavaScript variables can hold many different types of data:
1. String – Represents textual data (e.g., ;’Hello, World!’>).
2. Number – Represents both integer and floating-point numbers (e.g., ;42>, ;3.14>).
3. Boolean – Represents logical values, true or false.
4. Undefined – Represents a variable that has been declared but not yet assigned a value.
5. Null – Represents a deliberate non-value.
6. Object – Used to store collections of data and more complex entities.
7. Symbol – Represents unique identifiers for object properties.
Operators for Manipulating Data
JavaScript supports various operators for performing tasks like arithmetic operations, assignment to variables, comparing values, and more. Here are a few basic operator categories:
Arithmetic Operators
Perform mathematical calculations:
– Addition (;+>)
– Subtraction (;->)
– Multiplication (;*>)
– Division (;/>)
Assignment Operators
Assign values to variables:
– Basic assignment (;=>)
– Addition assignment (;+=>)
– Subtraction assignment (;-=>)
Comparison Operators
Compare two values and evaluate to true or false:
– Equal to (;==>)
– Strictly equal to (;===>)
– Greater than (;>>)
– Less than (;<>)
Understanding how to declare variables, knowing the types of data they can hold, and manipulating these values using operators are fundamental skills in JavaScript programming. As you become more familiar with these concepts, you’ll be able to create more complex and functional web applications. Remember, practice is key, so experiment with variables, data types, and operators to reinforce your learning.