PHP Debugging Strategies for Efficient Web Development
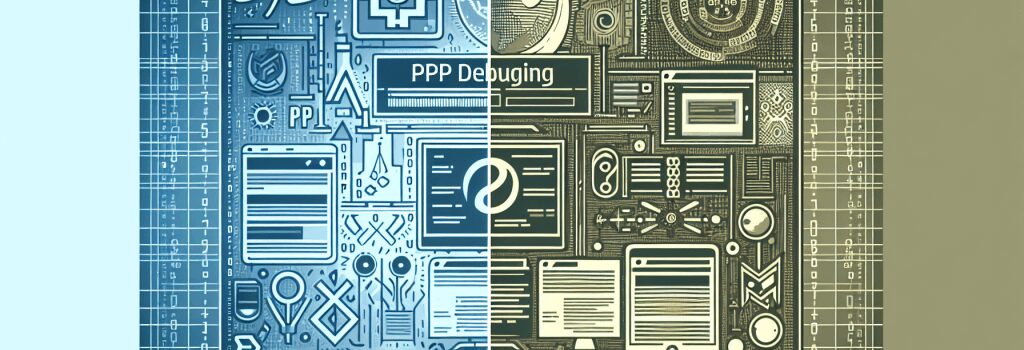
PHP debugging is a crucial step in the web development process, ensuring that your website runs smoothly and efficiently. Mastering the techniques of PHP debugging can significantly enhance your web development skills, making your websites more robust and error-free. In this article, we’ll walk you through some effective PHP debugging strategies designed to streamline your web development projects.
Understanding PHP Errors
Before diving into the debugging techniques, it’s important to understand the types of errors you might encounter in PHP. PHP errors can be broadly categorized into four types: Parse errors, Fatal errors, Warning errors, and Notice errors. Each type of error indicates a different issue, from syntax mistakes to attempting to access undefined variables. By familiarizing yourself with these errors, you can more quickly identify and resolve issues in your PHP code.
Setting Up a Local Development Environment
One of the best practices in PHP development is to set up a local development environment that mirrors your production environment. Tools like XAMPP or WAMP make it easy to develop and debug your applications locally before pushing changes live. This practice helps identify and resolve potential issues early in the development cycle, saving time and reducing the risk of deploying buggy code.
Utilizing PHP’s Built-in Error Reporting
PHP comes with built-in error reporting that can be incredibly helpful for identifying issues within your code. By modifying the ;php.ini> file or using the ;error_reporting()> function, you can configure PHP to display or log errors based on your current needs. For comprehensive debugging, it’s recommended to enable ;E_ALL> reporting, which displays all types of errors and warnings.
Debugging with Var_dump() and Print_r()
Simple yet powerful, ;var_dump()> and ;print_r()> are functions that allow you to print the contents of a variable to the browser. These functions are particularly useful for inspecting array or object values at various stages of your application’s execution. However, remember that continuously printing values to the browser can be cumbersome in larger applications, which is where more sophisticated debugging tools come into play.
Leveraging Xdebug for Advanced Debugging
Xdebug is a PHP extension that provides a range of debugging and profiling capabilities beyond PHP’s built-in functions. With Xdebug, you can step through your code line by line, inspect variable values at any point during execution, and analyze call stacks. This level of insight is invaluable for identifying hard-to-find bugs and performance bottlenecks in your applications.
Implementing Unit Testing with PHPUnit
Unit testing is a methodical approach to validating that individual units of code (such as functions or methods) work as expected. PHPUnit is a popular unit testing framework for PHP that can automate the testing process, ensuring that your code behaves correctly in various scenarios. Incorporating unit testing into your development workflow can significantly improve code quality and reliability.
Conclusion
Debugging is an integral part of web development that requires patience, practice, and the right tools. By understanding PHP errors, setting up a local development environment, utilizing PHP’s built-in error reporting, exploring simple functions like ;var_dump()> and ;print_r()>, leveraging advanced tools like Xdebug, and implementing unit testing with PHPUnit, you can tackle PHP debugging with confidence. These strategies will not only help you develop more efficiently but also contribute to building more stable, high-quality web applications.