Posts with tag “JavaScript”
JavaScript is a powerful and essential programming language for web development. In this tag, you will find a collection of posts dedicated to helping you learn and master JavaScript. From the basics of variables and functions to more advanced concepts like DOM manipulation and asynchronous programming, these posts will guide you through everything you need to know to become proficient in JavaScript and enhance your skills as a web developer.
Read more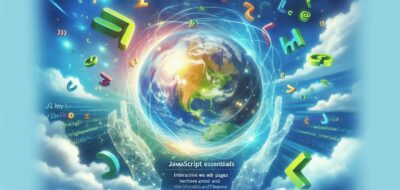
JavaScript Essentials: Interactive Web Pages and Beyond
--- JavaScript Essentials: Unlocking the Power of Interactive Web Pages and Beyond JavaScript has ...
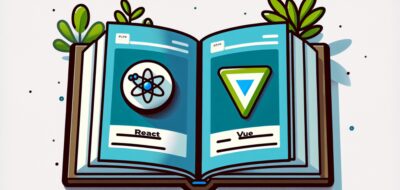
JavaScript Frameworks: An Introduction to React and Vue
JavaScript Frameworks: An Introduction to React and VueJavaScript has undeniably become a pivotal element ...
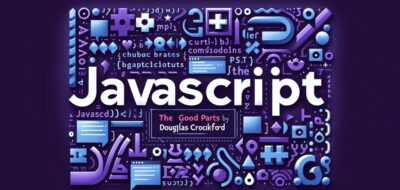
JavaScript: The Good Parts by Douglas Crockford
Unveiling the Essence of JavaScript with Douglas Crockford's MasterpieceIn the evolving landscape of web ...
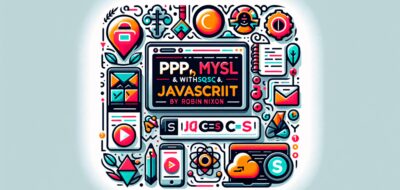
Learning PHP, MySQL & JavaScript: With jQuery, CSS & HTML5 by Robin Nixon
Master Web Development with a Comprehensive GuideIn the ever-evolving landscape of web development, having ...
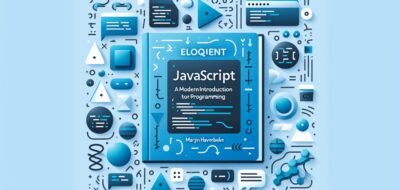
Eloquent JavaScript: A Modern Introduction to Programming by Marijn Haverbeke
Eloquent JavaScript: An Essential Guide for Aspiring Web DevelopersIn the journey of becoming a ...
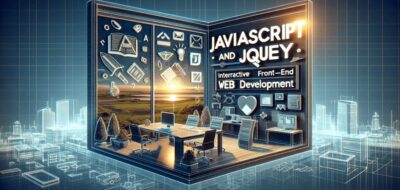
JavaScript and JQuery: Interactive Front-End Web Development by Jon Duckett
Unveiling the Power of Interactive Front-End Web Development: A Dive into JavaScript and jQuery ...
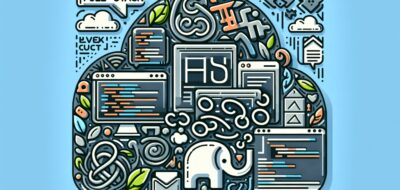
Full Stack Web Development: Integrating HTML, CSS, JavaScript, and PHP
Unlocking the Potential of Full Stack Web DevelopmentIn the swiftly evolving landscape of web ...