Variables and Scope in JavaScript: A Detailed Overview
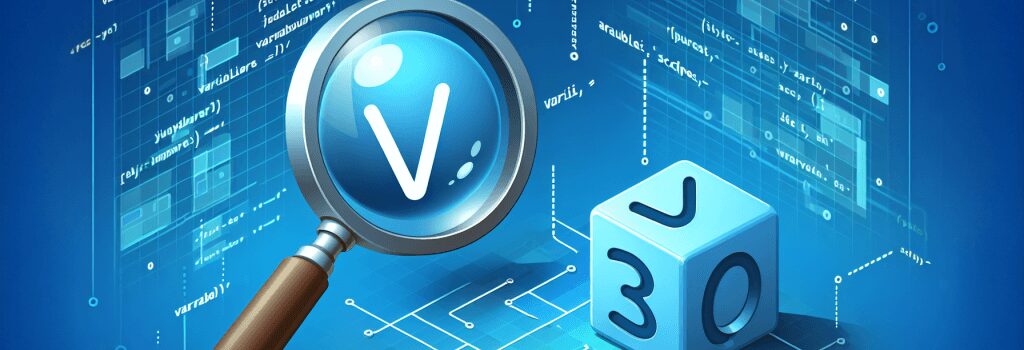
Understanding Variables and Scope in JavaScript
Variables play a crucial role in any programming language, and JavaScript is no exception. They are the backbone that allows developers to store, manipulate, and retrieve data within their applications. Combined with an understanding of scope, variables empower developers to write efficient, effective code. In this detailed overview, we’ll dive into the intricacies of variables and scope in JavaScript, shedding light on their importance and how they can be effectively utilized in your web development projects.
What Are Variables in JavaScript?
Variables in JavaScript are containers for storing data values. In JavaScript, a variable can be declared using three keywords: ;var>, ;let>, or ;const>. Each provides a different way of managing the data stored in it and comes with its own set of rules regarding scope and reassignment.
– var: Declares a variable, optionally initializing it to a value.
– let: Declares a block-scoped, local variable, optionally initializing it to a value.
– const: Declares a block-scoped, read-only named constant.
Data Types in JavaScript
Before delving further into variables, it’s essential to understand the different data types that can be stored in these containers. JavaScript supports several primitives, such as:
– Number: Represents both integer and floating-point numbers.
– String: Represents textual data.
– Boolean: Represents a logical entity that can have two values: true or false.
– Undefined: Indicates that a variable has not been assigned a value.
– Null: Represents the intentional absence of any object value.
– Symbol: Represents a unique identifier.
In addition to these primitives, JavaScript also supports objects, which can be thought of as collections of properties.
Understanding Scope in JavaScript
Scope determines the accessibility of variables and functions in different parts of your code. In JavaScript, there are two main types of scope:
– Global Scope: When a variable is declared outside any function, it becomes globally accessible. It can be accessed and modified from any part of the code.
– Local Scope: Contrary to global scope, variables declared within a function are locally scoped. They can only be accessed and modified within the function they are declared in. Local scope can further be divided into:
– Function Scope: Variables declared with ;var> within a function.
– Block Scope: Introduced in ES6, variables declared with ;let> and ;const> within any block ({…}) are scoped to that block.
Best Practices for Using Variables and Scope
To write clean, maintainable JavaScript code, it’s important to follow best practices regarding variables and scope:
1. Prefer ;let> and ;const> over ;var>: They provide block scope and reduce the risk of variable redeclaration and other scope-related bugs.
2. Minimize Global Variables: Too many global variables can lead to conflicts and hard-to-track bugs.
3. Use Descriptive Variable Names: Names should reflect the value the variable represents, making your code easier to read and maintain.
4. Initialize Variables: Always initialize your variables. An uninitialized variable can lead to unexpected behavior.
Understanding the nuances of variables and scope is vital for any aspiring JavaScript developer. By mastering these concepts, you can ensure that your code is robust, efficient, and less prone to bugs. Embrace these practices in your web development journey, and you’ll find that building dynamic, interactive websites becomes a more streamlined and enjoyable process.