Variable Scope and Hoisting in JavaScript: Key Concepts for Developers
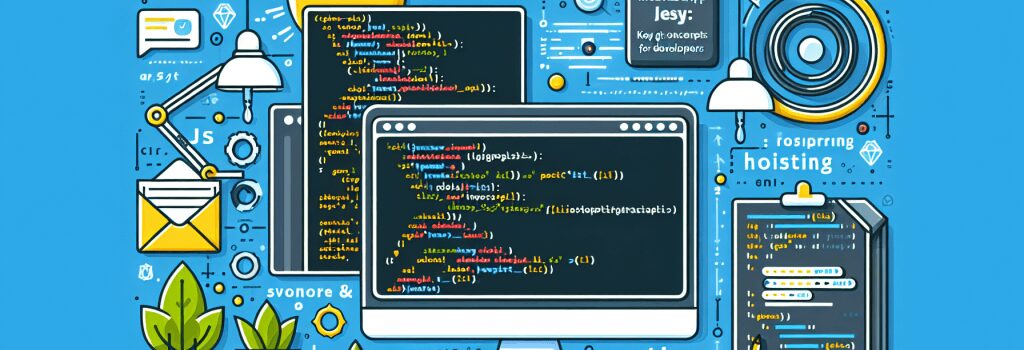
Understanding Variable Scope in JavaScript
For anyone venturing into the realm of web development, grasping the concept of variable scope in JavaScript is fundamental. Variable scope refers to the accessibility or visibility of variables in different parts of your code. This concept plays a crucial role in how variables are accessed, modified, and managed throughout your JavaScript programs.
Global vs. Local Scope
Variables in JavaScript can either have a global scope or a local scope. A globally scoped variable is declared outside of all functions and is accessible from any part of your code. On the other hand, locally scoped variables are declared within a function and can only be accessed within that function.
Global Scope Example:
Local Scope Example:
Understanding Hoisting in JavaScript
Hoisting is a JavaScript mechanism where variables and function declarations are moved to the top of their containing scope before code execution. Regardless of where variables and functions are declared, they are hoisted to the top of their scope, allowing them to be used before they are actually declared.
Var, Let, and Const
The behavior of hoisting depends on how a variable is declared. In JavaScript, variables can be declared using ;var>, ;let>, or ;const>.
– Var: Variables declared with ;var> are hoisted to the top of their functional or global scope and initialized with ;undefined>.
– Let and Const: Variables declared with ;let> or ;const> are hoisted to the top of their block scope, but are not initialized. Accessing them before their declaration results in a ;ReferenceError>.
Hoisting Example:
Best Practices
Understanding variable scope and hoisting is crucial for writing efficient and error-free JavaScript code. Here are some best practices to follow:
– Minimize the use of global variables to avoid unexpected behavior in larger applications.
– Use ;let> or ;const> for variable declarations to take advantage of block-scoping and reduce hoisting issues.
– Declare variables at the beginning of their scope, as close as possible to their first use to make your code more readable and to avoid confusion related to hoisting.
By mastering these key concepts and adhering to best practices, you’ll be better equipped to develop robust and error-free JavaScript code, paving your way to becoming a proficient web developer.