Utilizing CSS in JavaScript for Dynamic Styling
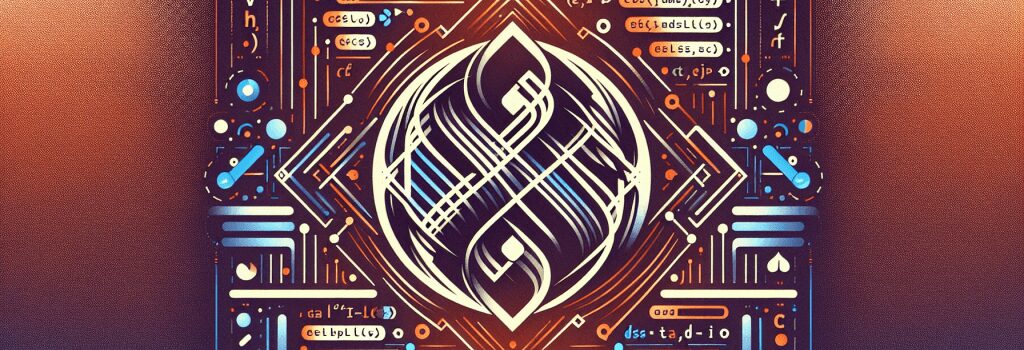
Utilizing CSS in JavaScript for Dynamic Styling
In the fast-paced world of web development, the ability to dynamically adjust the style of web elements is crucial for creating interactive and user-responsive sites. JavaScript, coupled with Cascading Style Sheets (CSS), offers a powerful toolkit for developers to enhance the user experience with dynamic styling. This article delves into methods and best practices for utilizing CSS in JavaScript, a fundamental skill for anyone aiming to become a proficient web developer.
Understanding the Basics
Before diving into the intricate details of manipulating CSS with JavaScript, it’s essential to have a firm grasp of both these technologies. CSS is used for styling HTML elements, determining their appearance and layout on a web page. JavaScript, on the other hand, is a programming language that allows you to implement complex features on web pages, including interacting with CSS to change styles dynamically.
Inline CSS with JavaScript
One of the simplest ways to change the style of an element using JavaScript is by manipulating inline CSS. This method involves altering the ;style> property of an HTML element directly.
Example:
This code snippet changes the text color of the element with the ID ;myElement> to blue. Such direct manipulation is straightforward but can quickly become cumbersome for multiple style changes or complex applications.
Using ;className> and ;classList>
To manage multiple styles efficiently, JavaScript provides two properties: ;className> and ;classList>.
The ;className> Property
The ;className> property allows you to set or update the class attribute of an HTML element.
Example:
This replaces any existing classes of ;myElement> with the "highlight" class, assuming you have the appropriate CSS rules defined.
The ;classList> Property
;classList> is a more powerful feature that offers methods like ;add()>, ;remove()>, and ;toggle()> for more control over an element’s classes.
Example:
This simply adds the "highlight" class without affecting other existing classes, making ;classList> preferable for adding or removing specific styles dynamically.
Manipulating CSS with JavaScript for Interaction
Dynamic styling becomes particularly useful when dealing with user interactions. Consider a scenario where you want to change the appearance of a button when it’s clicked. JavaScript can handle the event and modify the button’s CSS, providing immediate visual feedback to the user.
Example:
In this example, clicking the button toggles the "active" class, enabling developers to enhance interactivity with simple CSS transitions or animations tied to these class changes.
Best Practices for Dynamic Styling
When utilizing CSS in JavaScript for dynamic styling, consider the following best practices to maintain readability, performance, and maintainability of your code:
– Use meaningful class names: Choose class names that reflect their purpose or the style they represent, making your code easier to understand.
– Limit direct style manipulations: Prefer manipulating classes with ;className> or ;classList> over direct inline style changes to keep your JavaScript cleaner and more maintainable.
– Externalize CSS: Keep your styles in external CSS stylesheets rather than embedding them in JavaScript, facilitating easier styling updates and code separation.
Conclusion
Combining CSS with JavaScript to achieve dynamic styling is a powerful technique in web development. By understanding and utilizing methods like inline styles, ;className>, and ;classList>, developers can create more interactive, responsive, and engaging web applications. Following best practices ensures that your code remains clean, performant, and maintainable as you incorporate dynamic styling into your projects.