Understanding JavaScript Functions: A Beginner’s Guide
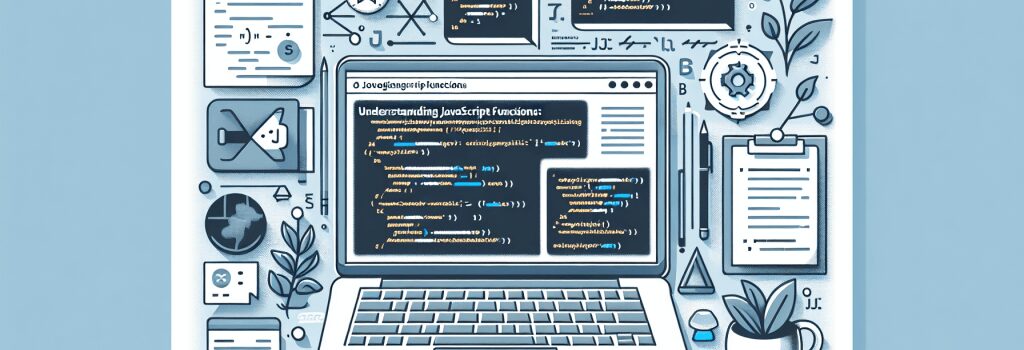
Introduction to JavaScript Functions
JavaScript functions are one of the building blocks of web development. They allow you to encapsulate reusable code, making your JavaScript more efficient and your web applications more dynamic. Understanding how to use functions is essential for anyone looking to become a proficient web developer. This guide aims to explain functions in JavaScript in a clear and straightforward manner, perfect for beginners.
What is a JavaScript Function?
A function in JavaScript is a set of statements that performs a task or calculates a value. Functions allow you to package up lines of code that you can use (and often reuse) throughout your scripts. Functions can take inputs, called parameters, and can return a value.Creating Your First Function
The basic syntax for a function is:For example, a simple function to add two numbers would look like this:
You can call or invoke this function by using its name followed by parentheses, including any required parameters:
Understanding Function Parameters and Arguments
Parameters are names listed in the function’s definition. Arguments are the real values passed to (and received by) the function. In the example above, ;x> and ;y> are parameters, while ;5> and ;10> are the arguments passed to the function.
Types of Functions
– Named Functions: The example above is a named function, identified by the name ‘add’.– Anonymous Functions: Functions without a name. These are often used as arguments to other functions or as Immediately Invoked Function Expressions (IIFEs).
– Arrow Functions: Introduced in ES6, arrow functions provide a concise syntax for writing functions in JavaScript. They are especially handy when working with callbacks or methods like map, reduce, or filter on arrays.
Arrow Function Example
The Role of Functions in Web Development
Functions play a crucial role in web development, allowing developers to:
– Manage and organize code efficiently.
– Implement logic and algorithms required for the functionality of web applications.
– Respond to events such as mouse clicks, form submissions, and page loads.
– Manipulate data, control the Document Object Model (DOM), and interact with web APIs.
Best Practices when Working with JavaScript Functions
– Naming Conventions: Choose clear, descriptive names for your functions.– Single Responsibility: Each function should do one thing well. If a function performs multiple tasks, consider breaking it into smaller functions.
– Keep It DRY (Don’t Repeat Yourself): Reuse functions to avoid duplicating code.
– Documentation: Comment your functions to explain what they do, what parameters they accept, and what they return.