Traversing the DOM: Techniques and Tips
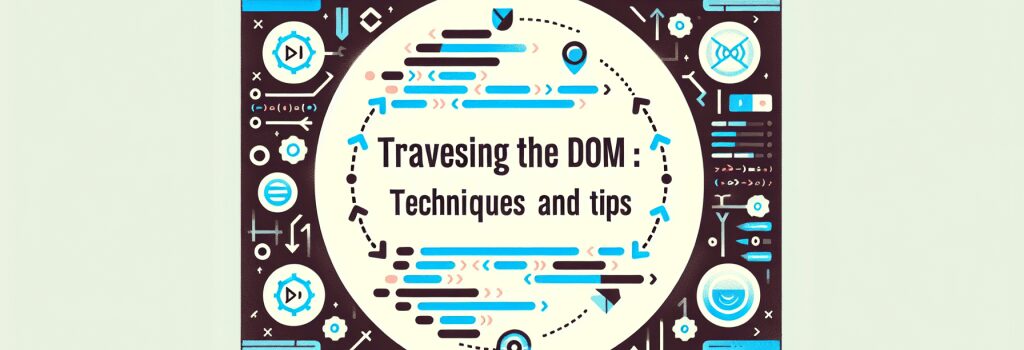
Traversing the DOM: Techniques and Tips
The Document Object Model (DOM) is a crucial aspect of web development, serving as the bridge between your code and what is displayed on the browser. Effective manipulation of the DOM allows developers to create dynamic and interactive web pages. Understanding how to traverse the DOM efficiently is essential for any aspiring web developer. In this article, we’ll delve into various techniques and tips for traversing the DOM using JavaScript, ensuring you have the knowledge to enhance your web development projects.
Understanding the DOM Tree
Before we jump into traversing techniques, it’s important to grasp the structure of the DOM. The DOM represents the webpage as a hierarchical tree of nodes, including elements, text, and attributes. Each node is connected, representing parent-child and sibling relationships, making navigation through the tree possible.
Basic Traversing Techniques
Selecting Individual Elements
– getElementById: A method to select a singular element by its ID. It is a straightforward way to access a specific element directly.
– querySelector: Allows you to select the first element that matches a CSS selector. It provides more flexibility than ;getElementById> since you can use it to select classes, tags, and much more.
Selecting Multiple Elements
– getElementsByTagName: This method selects all elements of a specific tag name and returns a live HTMLCollection. It’s helpful for applying changes to all elements of the same tag.
– getElementsByClassName: Similar to ;getElementsByTagName>, but selects elements by their class name.
– querySelectorAll: Returns a static NodeList containing all elements that match a specified CSS selector. Unlike ;getElementsByTagName> and ;getElementsByClassName>, ;querySelectorAll> provides a snapshot of elements, allowing for more complex selections.
Navigating Between Elements
Understanding how to move around the DOM tree is just as important as being able to select elements. Here are some properties used for navigating between elements:
Parent, Child, and Sibling Relationships
– parentNode: Access the parent node of the current element.
– childNodes: Access all child nodes of an element, including text and comment nodes.
– children: Similar to ;childNodes>, but returns only the element children, ignoring text and comment nodes.
– firstChild and lastChild: Access the first and last child node of an element respectively.
– nextSibling and previousSibling: Navigate between siblings of the current node, moving forward or backward.
Tips for Efficient DOM Traversal
1. Use Specific Selectors: Whenever possible, use the most direct selector to access an element. This reduces the need to traverse the DOM and increases the efficiency of your code.
2. Minimize DOM Access: Accessing the DOM can be costly in terms of performance. Minimize the number of times you access the DOM by storing references to elements in variables when they need to be accessed multiple times.
3. Understand the Cost of Live Collections: Methods like ;getElementsByTagName> return live HTMLCollections which update dynamically. This can be useful but also expensive in terms of performance. Use static collections like those returned by ;querySelectorAll> for better performance in certain scenarios.
4. Leverage Document Fragments: When making multiple changes to the DOM, consider using a document fragment to assemble your changes and append the fragment to the document in a single operation. This can significantly reduce page reflow and improve performance.
By mastering these techniques and tips, you’ll be well-equipped to navigate and manipulate the DOM effectively. Traversing the DOM is a foundational skill for any web developer, enabling the creation of rich, interactive user experiences. Remember, the key to becoming proficient in DOM manipulation is practice, so don’t hesitate to experiment with these techniques in your projects.