The Role of Objects in JavaScript: Properties, Methods, and Prototypes
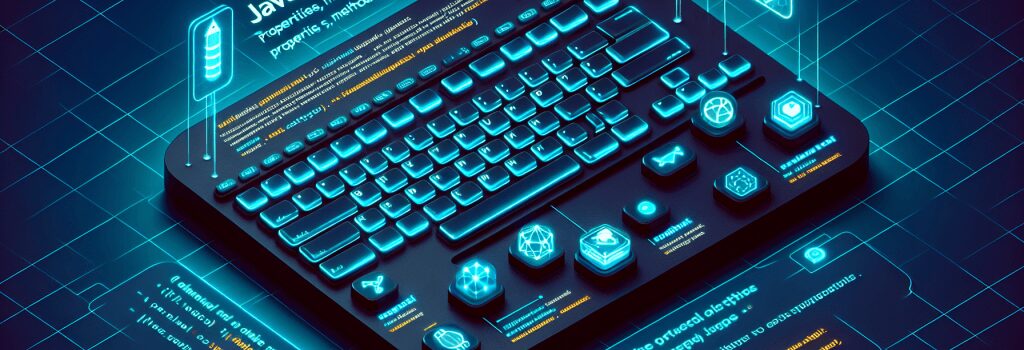
Understanding the Role of Objects in JavaScript: A Comprehensive Guide
Introduction
JavaScript, as a core technology of the web, plays a fundamental role in modern web development. Mastering JavaScript means understanding its foundational concepts, including the pivotal role of objects. Objects in JavaScript are incredibly versatile, serving as the backbone for more complex data structures, offering a framework for organizing code, and providing a gateway to object-oriented programming principles.
What Are Objects in JavaScript?
Objects in JavaScript are collections of related data and functionality. They are used to store, manipulate, and convey data in a structured manner. At their core, objects consist of properties and methods:
– Properties are values associated with an object. These values can be strings, numbers, or even functions.
– Methods are functions that belong to an object, and they typically describe actions that an object can perform.
Objects can be created in multiple ways, but perhaps the most straightforward method is using object literals, defined by enclosing a comma-separated list of key-value pairs within curly braces ;{}>.
Understanding Properties
Properties in JavaScript objects can be accessed and manipulated in several ways. The dot notation (;object.property>) is perhaps the most common, but bracket notation (;object[“property”]>) is invaluable when dealing with dynamic property names or properties that contain characters not allowed in variable names.
Dive into Methods
Methods are essentially properties that store function values. These functions can access and modify an object’s data, leveraging the ;this> keyword to reference the object itself. Methods play a crucial role in encapsulating functionality within an object, promoting code reusability and maintainability.
Exploring Prototypes
JavaScript employs prototypes as a means of inheritance, allowing objects to inherit properties and methods from other objects. Every JavaScript object has a ;prototype> property, which links to another object. This linked object can have its own prototype, creating a “prototype chain” that JavaScript uses to look up properties and methods.
Prototype-Based Inheritance
Prototype-based inheritance is a fundamental JavaScript concept where objects can inherit properties and methods from other objects. By understanding prototypes, developers can create complex hierarchical structures that mimic classical inheritance, albeit in a dynamic and flexible manner unique to JavaScript.
Conclusion
In the multifaceted world of web development, understanding the role of objects in JavaScript is indispensable. Objects, with their properties and methods, offer a structured approach to managing data and functionality. Prototypes introduce a powerful, albeit sometimes challenging, layer of flexibility and reuse in code design. For anyone aspiring to become a proficient web developer, mastering JavaScript objects is a crucial milestone on the path to leveraging the full potential of this ubiquitous programming language.
By delving into these topics, developers not only gain a deeper understanding of JavaScript but also equip themselves with the foundational knowledge required to explore more advanced topics and frameworks. Embrace the journey of learning and let the robust structure of JavaScript objects guide your path to becoming a versatile and efficient web developer.