The Basics of Adding Event Listeners in JavaScript
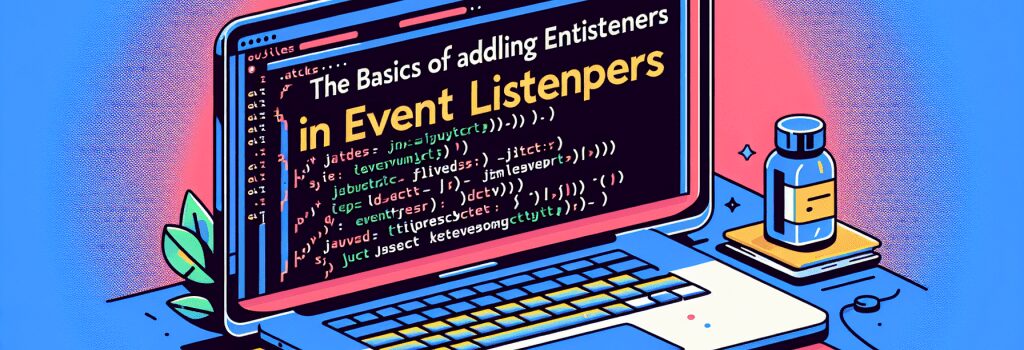
Introduction to Event Listeners in JavaScript
Event listeners in JavaScript are powerful tools that enable developers to create dynamic and interactive web applications. By understanding how to use event listeners effectively, you can respond to user actions such as clicks, keyboard inputs, and page loads, making your web applications more engaging and user-friendly.
Understanding Event Listeners
Event listeners are essentially JavaScript functions that listen for events on web page elements. When the specified event occurs, the event listener triggers a callback function, executing the code you’ve defined to respond to the event.
How Event Listeners Work
1. Select the Element: Before you can add an event listener, you need to select the DOM (Document Object Model) element you want to listen to. This can be done using methods like ;document.getElementById()>, ;document.querySelector()>, or similar DOM selection methods.
2. Define the Event Type: JavaScript supports various event types, including ;click>, ;mouseover>, ;keydown>, ;load>, and many others. You’ll need to specify the type of event you want your event listener to respond to.
3. Create the Callback Function: This function contains the code that will execute when the event occurs. It can be as simple or complex as your application requires.
4. Add the Event Listener: Use the ;addEventListener()> method to attach the event listener to your selected element, specifying the event type and callback function.
Adding an Event Listener: A Step-by-Step Guide
Step 1: Selecting the Element
Step 2: Defining the Callback Function
Step 3: Adding the Event Listener
Best Practices for Using Event Listeners
– Use Descriptive Function Names: Choose function names that clearly describe their purpose, making your code more readable and maintainable.
– Utilize Anonymous Functions for Simple Actions: For simple event responses, consider using anonymous functions directly in your ;addEventListener()> call.
– Consider Event Propagation: Be aware of event propagation (bubbling and capturing) and how it affects your event listeners. Use ;event.stopPropagation()> when needed to prevent undesired behavior.
– Remove Event Listeners When Not Needed: To optimize performance and prevent memory leaks, remove event listeners that are no longer necessary using the ;removeEventListener()> method.
Conclusion
Mastering the basics of adding event listeners in JavaScript is a crucial step towards becoming a proficient web developer. By effectively utilizing event listeners, you can create interactive and responsive web applications that provide a seamless user experience. Remember to follow best practices and always look for ways to enhance the user’s interaction with your web pages. Happy coding!