Strategies for Efficiently Handling Multiple AJAX Calls in JavaScript
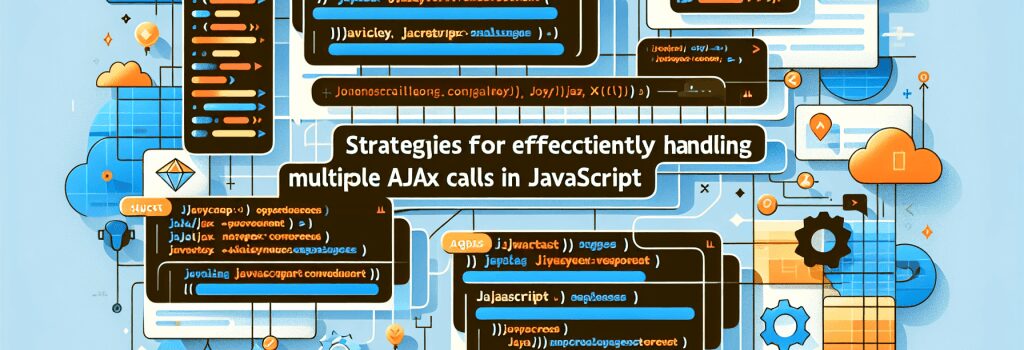
The digital realm awaits you, brave adventurer, which is filled with quests and encounters that involve AJAX calls (or Asynchronous JavaScript and XML, but that’s a mouthful!). In this chapter, we’re going to explore how you, a novice web developer, can streamline your approach at tackling a hydra of AJAX calls in JavaScript with finesse and aplomb.
Remember, as a web developer, your job is to serve your users the information they need when they need it, not when the system gets around to it. So, let’s get on with it.
The AJAX Call Jumble
Imagine you are at a busy restaurant where your order depends on the orders of everyone else. This situation would be chaotic, right?
The same case applies to managing multiple AJAX calls in JavaScript. If AJAX calls are not handled efficiently, it’s like a restaurant with one chef catering to all tables. But worry not, our digital cuisine offers some delicious solutions.
Sequential AJAX Calls
Sequential AJAX calls are the equivalent of the afore-mentioned chef finishing up one order before moving to the next. Now, this isn’t necessarily a bad thing. It’s straightforward: ‘take order, cook, serve, repeat.’ Simple, Right? But not always efficient.
Here’s a code snippet to show sequential handling:
This approach works, but not if you have customers (users) waiting for their ‘orders’ at the same time.
Concurrent AJAX Calls
Now, let’s up the game by introducing some sous-chefs. Concurrent AJAX calls are like dealing with several orders at once. Here’s how you can manage concurrent AJAX calls:
In this scenario, all AJAX calls are made concurrently, and the Promise.all waits until all promises are fulfilled before executing the final code.
Parallel AJAX Calls
Why stop at concurrent when you can go parallel? Parallel AJAX calls utilize web workers – think of them as chefs specialized in different styles of cooking. Each AJAX call is handled by a different web worker.
Sadly, due to the space constraint (and because our restaurant doesn’t have a Michelin star yet), we aren’t going to delve into a detailed code analysis of parallel AJAX calls.
Choosing the Right Strategy
Choosing the right AJAX call strategy is crucial. It’s akin to selecting the right ingredients for a dish.
Sequential calls are great when calls depend on the results of a previous call.
Concurrent calls work best when the calls are independent, but you need to wait for all to complete.
* Parallel calls are excellent for heavy, CPU-intensive AJAX calls where distribution of tasks offers advantage.
Keep practicing, aspiring chefs! Soon enough, the digital broth of AJAX calls will be your specialty. Remember, in the world of web development, there’s always a strategy to suit your needs, it’s just a matter of finding it.
Here ends our adventurous AJAX journey. Now, go forth and code!