Performance Optimization Tips for JavaScript and AJAX in Web Development
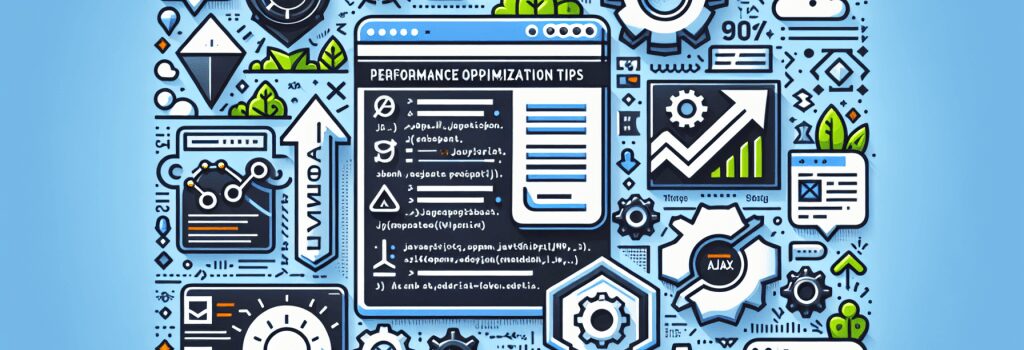
Understanding Performance in JavaScript and AJAX
In the realm of web development, performance is key to delivering smooth, fast, and responsive user experiences. JavaScript and Asynchronous JavaScript and XML (AJAX) are central to this endeavor, being at the heart of modern web applications. However, poorly optimized JavaScript and AJAX can lead to sluggish interfaces. Let’s delve into how you can optimize your JavaScript and AJAX code for peak performance.
Efficient JavaScript for Web Developers
Minimize and Compress Your Code
One of the first steps in optimizing JavaScript is minimizing and compressing your code. Tools like UglifyJS and Terser can remove unnecessary characters (such as whitespace, comments, etc.) from your code without changing its functionality. Compression reduces file size, leading to shorter download times and faster execution.
Leverage Browser Caching
Browser caching can significantly improve performance for returning visitors. By caching static resources like JavaScript files, you can reduce server requests, leading to quicker page loads. Ensure your server is correctly configured to allow adequate caching of these resources.
Asynchronous Loading
Loading scripts asynchronously ensures that they do not block the rendering of your page. This means your webpage can load and become interactive more quickly. Utilize the ;async> or ;defer> attributes when including JavaScript files to prevent blocking.
Optimizing AJAX Requests
Reducing the Number of Requests
Every AJAX request you make to a server carries overhead. Reduce the number of AJAX calls by combining requests where possible and ensuring that only necessary data is being requested. This reduces latency and server load, leading to faster response times.
Using GET Requests for Data Fetching
When fetching data that doesn’t require server-side processing, use GET requests. GET requests can be cached by the browser, unlike POST requests, which can improve performance for frequently accessed resources.
Handling Data Efficiently
When you receive data from an AJAX call, handling it efficiently is crucial. Parse and process data seamlessly to prevent UI glitches. If you’re working with large datasets, consider implementing infinite scrolling or pagination to reduce the initial load time.
JavaScript and AJAX Best Practices
Debouncing and Throttling
Debouncing and throttling are techniques to limit the number of times a function is executed. Debouncing is particularly useful for events that trigger many times (e.g., window resizing), ensuring the function runs only after a certain amount of time. Throttling ensures a function is only executed at every specified time interval. Both techniques can dramatically enhance performance when used appropriately.
Use Web Workers for Complex Calculations
JavaScript runs in a single thread, which means compute-heavy tasks can block the UI, leading to poor user experiences. Web Workers allow you to run JavaScript in background threads, thereby offloading heavy computations and keeping your UI responsive.
Efficient DOM Manipulation
Direct manipulation of the Document Object Model (DOM) can be expensive. Minimize direct DOM manipulation, use virtual DOM where possible, and batch your updates to avoid performance bottlenecks.
Conclusion
Optimizing JavaScript and AJAX requests is crucial for creating fast, responsive web applications. By applying these strategies, developers can improve the performance of their web applications, resulting in better user experiences. Remember, performance optimization is an ongoing process, and staying informed about best practices in JavaScript and AJAX is key to maintaining and improving the quality of your web applications.