Optimizing Your JavaScript Code for Efficient Event Handling and AJAX Requests
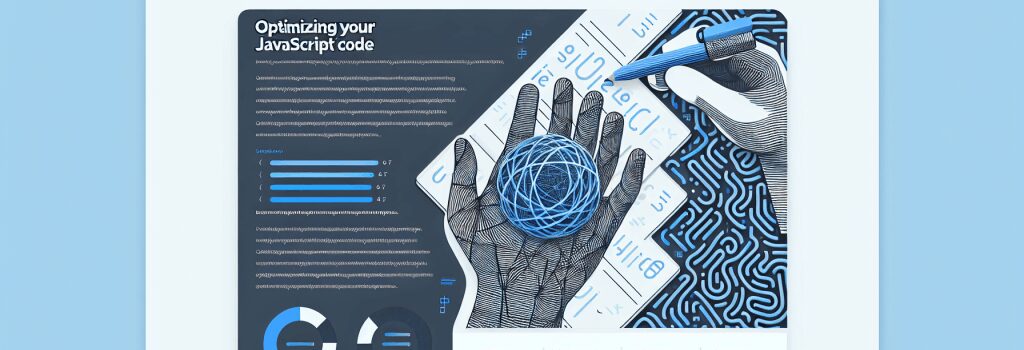
Effective Strategies for JavaScript Code Optimization in Event Handling and AJAX Requests
In the world of web development, creating interactive and dynamic user interfaces heavily relies on proficient event handling and seamless AJAX requests. JavaScript stands as a cornerstone technology in this endeavor. This article delves into optimizing your JavaScript code to ensure your web applications run efficiently, focusing on event handling and AJAX requests.
Understanding the Basics of Event Handling and AJAX in JavaScript
Event Handling in JavaScript is pivotal for creating responsive and interactive web pages. It involves writing functions called event handlers that execute when a specific event, like a click or a keystroke, occurs. To optimize event handling:
– Use Event Delegation: Instead of attaching event listeners to each element, use a single listener on a parent element. This reduces memory consumption and enhances performance.
– Throttle and Debounce Events: When dealing with events that trigger numerous times within a short span, like resizing or scrolling, applying throttling or debouncing techniques minimizes the number of times the event handler is called, thereby easing the workload on the browser.
Asynchronous JavaScript and XML (AJAX) allows web pages to communicate with a server asynchronously, fetching data without reloading the page. For efficient AJAX requests:
– Minimize DOM Manipulations: Each manipulation can affect performance. Batch updates or use Document Fragments to minimize reflows and repaints.
– Use the Fetch API: Modern and more powerful than the traditional ;XMLHttpRequest>, the Fetch API supports promises, making it easier to write cleaner, more readable asynchronous code.
Optimizing JavaScript Performance for Event Handling and AJAX
Efficiently Handling JavaScript Events
Set Passive Event Listeners: By marking an event listener as passive (;{passive: true}>), you tell the browser that the event doesn’t prevent default behavior. This is especially useful for touch and wheel events, improving scrolling performance on mobile devices.
Leveraging AJAX Requests for Peak Performance
Strategic Use of Caching: Implement caching strategies to store AJAX responses. It reduces server requests for the same data, significantly speeding up response times for your users.
Monitoring and Debugging
Leverage Browser DevTools: Modern browsers come equipped with powerful tools for monitoring JavaScript performance, including event handling and AJAX requests. Use these to identify bottlenecks and optimize accordingly.
Best Practices for Clean and Efficient Code
– Keep Your Code DRY (Don’t Repeat Yourself): Reuse code with functions to keep your JavaScript clean and less error-prone.
– Code Splitting: Divide your JavaScript code into smaller, more manageable chunks. This improves load times by only serving the code users need when they need it.
– Minify and Compress: Minify your JavaScript code and use compression techniques like Gzip to decrease file size, resulting in faster download and execution times.
Conclusion
Incorporating these optimization techniques into your JavaScript programming can significantly enhance the performance of your web applications, particularly in the realms of event handling and AJAX requests. Remember, the goal is to provide a smooth, seamless user experience without sacrificing functionality or design. By following the strategies outlined above, developers can write more efficient, maintainable, and faster web applications, ensuring a pleasant experience for end-users and a rewarding development process.
Empower your web development with optimized JavaScript code, and create web applications that not only meet but exceed user expectations. The journey towards efficient web development is continuous, and staying informed and adaptable with your optimization practices is key to success.