Mastering If-Else Statements in JavaScript
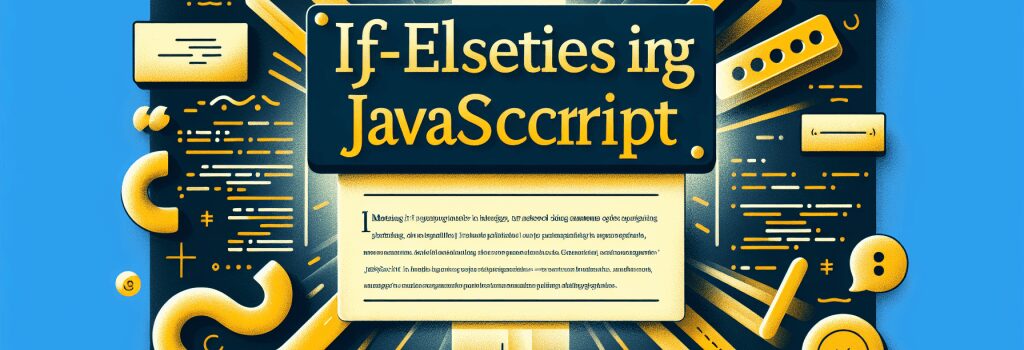
Mastering If-Else Statements in JavaScript
Introduction
In the world of web development, understanding the basics of JavaScript is crucial for creating interactive websites. A fundamental concept that every aspiring web developer must grasp is the use of control flow and conditional statements, particularly the ;if-else> statement. This allows your programs to make decisions based on certain conditions, leading to dynamic and responsive web applications. In this comprehensive guide, we’ll dive deep into mastering ;if-else> statements in JavaScript, breaking down how they work and providing practical examples to solidify your understanding.
Understanding If-Else Statements
What is an If-Else Statement?
An ;if-else> statement is a basic programming construct that executes a block of code if a specified condition is true. If the condition is false, it can execute an alternative block of code. This simple yet powerful tool is fundamental in making decisions in your code.
The Syntax of If-Else Statements
An ;if-else> statement in JavaScript is written as follows:
The condition within the parentheses is evaluated. Based on this evaluation, either the code within the first set of curly braces runs (if the condition is true) or the code within the ;else> block runs (if the condition is false).
Using If-Else Statements
Simple Example
Let’s look at a straightforward example to understand how ;if-else> statements operate:
In this example, if the variable ;age> is greater than 18, the message "You are eligible to vote." gets logged to the console. Otherwise, the message "You are not eligible to vote." is displayed.
Handling Multiple Conditions
<h4>The If-Else If StatementFor situations where you need to evaluate multiple conditions, JavaScript provides the ;if-else if> structure. This allows for more complex decision-making processes in your programs.
In this scenario, multiple conditions are checked in sequence. Once a true condition is found, the associated block of code is executed, and the rest are skipped.
Best Practices
– Keep it readable: Ensure your conditions are straightforward and understandable. Complex conditions can often be broken down or simplified.
– Avoid deep nesting: Deeply nested ;if-else> statements can make your code hard to follow. Consider alternative structures like ;switch> statements or using early returns.
– Use strict comparison: When evaluating conditions, prefer using ;===> and ;!==> over ;==> and ;!=> to avoid unexpected type coercion.
– Comment generously: Especially for complex conditions, comments can help others (and future you) understand the logic behind your decisions.
Conclusion
Mastering ;if-else> statements is an essential skill for any aspiring JavaScript developer. They are the backbone of decision-making in web development, enabling dynamic content and interactive user experiences. By understanding and applying the concepts outlined in this guide, you’ll be well on your way to building more sophisticated and responsive web applications. Remember, practice is key to becoming proficient, so experiment with ;if-else> statements in your projects to solidify your understanding.
Happy coding, future web developer!