Manipulating HTML Elements Using JavaScript
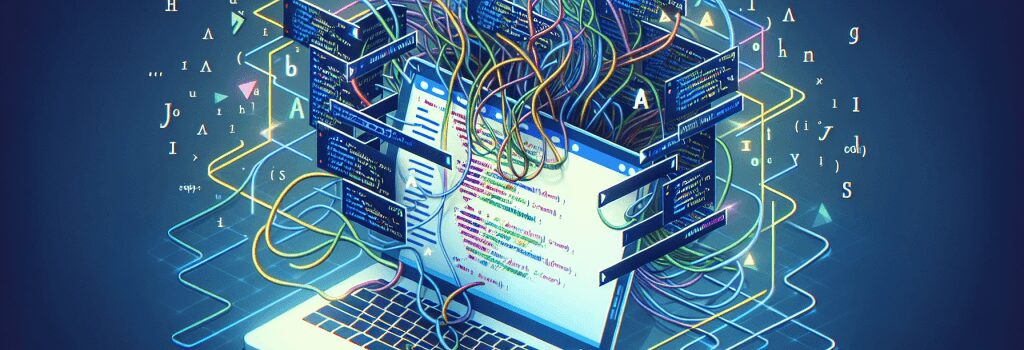
Manipulating HTML Elements Using JavaScript
In the modern web development ecosystem, JavaScript stands out as a core technology, enabling developers to create dynamic, interactive websites. Among its powerful features, the ability to manipulate the Document Object Model (DOM) is perhaps the most significant. This article delves into the essentials of DOM manipulation using JavaScript, guiding you through the process of enhancing your web pages by dynamically changing their content, style, and structure.
Understanding the DOM
Before diving into manipulation, it’s crucial to grasp what the DOM is. The Document Object Model is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content. The DOM represents the document as nodes and objects; this way, programming languages like JavaScript can interact with the page.
Selecting Elements
To manipulate an HTML element, you first need to select it. JavaScript provides several methods for this purpose:
getElementById
The ;document.getElementById()> method is one of the most common ways to select an element by its ID.
querySelector and querySelectorAll
For more complex selections, ;querySelector()> and ;querySelectorAll()> are invaluable. They allow you to select elements using CSS selectors.
Changing Element Content
Once you’ve selected an element, you can modify its content using the ;innerHTML> or ;textContent> properties.
innerHTML
The ;innerHTML> property lets you get or set the HTML content of an element.
New content
';textContent
The ;textContent> property allows you to set the text content of an element, without any HTML tags.
Modifying Styles
JavaScript enables you to alter the CSS styles of HTML elements dynamically. This is done by accessing the ;style> property of an element.
This method can apply any CSS styles, instantly reflecting on the page, allowing for interactive and responsive design elements to be implemented easily.
Adding or Removing Elements
Dynamic web applications often require adding or removing elements on the fly. JavaScript facilitates this through methods like ;appendChild()>, ;removeChild()>, and ;replaceChild()>.
Adding Elements
To add an element to the DOM, create the element first and then append it.
Removing Elements
To remove an element, select the parent element and then remove its child.
Conclusion
DOM manipulation with JavaScript is a fundamental skill for web developers, enabling the creation of dynamic, user-interactive web pages. By mastering selection methods, content modification, style changes, and adding or removing elements, you can take your web development projects to new heights. This tutorial offers a solid foundation, but the possibilities are vast, and continuous learning will uncover more advanced techniques and strategies for using JavaScript effectively in web development.
Embarking on the journey of mastering JavaScript and its applications in DOM manipulation will not only expand your skillset but also enhance the user experience of the web projects you undertake.