JavaScript Object Notation (JSON): Working with Data
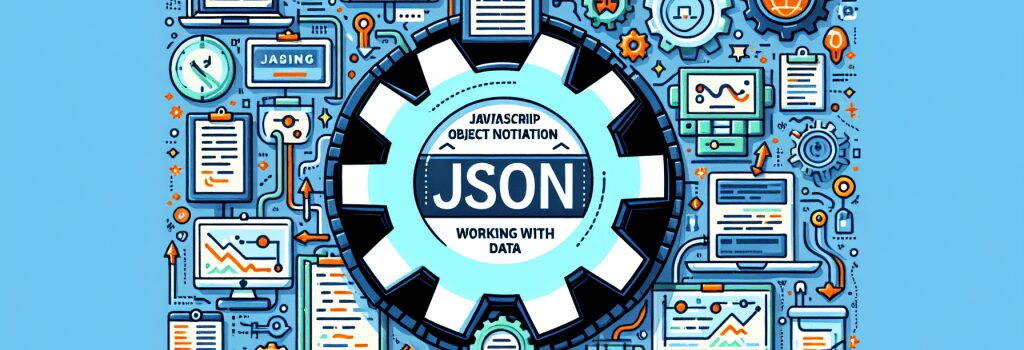
Understanding JavaScript Object Notation (JSON)
JavaScript Object Notation (JSON) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is based on a subset of the JavaScript Programming Language, Standard ECMA-262 3rd Edition – December 1999. JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others. This property makes JSON an ideal language for data interchange.
What is JSON?
JSON is a syntax for storing and exchanging data. It is text-written, using braces to hold objects, and square brackets to hold arrays. JSON is preferred for data interchange over XML, as it is shorter, easier to read, and easier to parse.
JSON Syntax in Detail
– Objects: JSON objects are surrounded by curly braces ;{}>. They are written in key/value pairs, keys being strings, and values being a valid JSON data type (string, number, object, array, boolean or null). Example: ;{"name": "John", "age": 30, "city": "New York"}>
– Arrays: JSON arrays are surrounded by square brackets ;[]> and contain a list of items. These items can be of any data type and can also include objects and arrays. Example: ;["Apple", "Banana", "Cherry"]>
– Data Types: JSON supports strings (text wrapped in double quotes), numbers (integers or floats), objects (a collection of key/value pairs), arrays (ordered lists of values), booleans (;true> or ;false>), and null.
Practical Applications of JSON in Web Development
Ajax Calls
One of the most common uses of JSON is to exchange data to/from a web server. When data is sent from a server to a web page, it can be easily converted from JSON to JavaScript objects for easy manipulation, making it perfect for asynchronous browser/server communication (Ajax).
Configuration Files
JSON format is widely used in configuration files for modern web applications and development tools. Its ability to naturally represent data structures makes it a straightforward choice for settings that need to be editable and readable.
APIs and Web Services
Web services and APIs use JSON format to provide public data. It is lightweight and easy to work with, making it ideal for transferring data between a server and a client.
Working with JSON in JavaScript
Parsing JSON
To work with JSON data in JavaScript, you might need to parse it. Parsing converts a JSON text to a JavaScript object. This can be done with the ;JSON.parse()> method.
Stringifying JSON
Conversely, if you have data in a JavaScript object and you want to send this data to a server, you will need to convert the object into a JSON string. This is done with the ;JSON.stringify()> method.
Conclusion
JSON has greatly simplified data interchange in web development, making it much more efficient and pleasant to work with asynchronous web technologies. Understanding how to work with JSON is essential for modern web developers, enabling seamless data exchange and manipulation across different systems.
Grasping JSON’s syntax, practical applications, and how to manipulate it within JavaScript lays a foundational skill for anyone aspiring to develop websites or work with web technologies. The simplicity and efficiency of JSON have made it the backbone of data interchange on the web, a crucial component of HTML, PHP, CSS, JS, and WordPress stacks in web development.