JavaScript Loops: Automating Repetitive Tasks
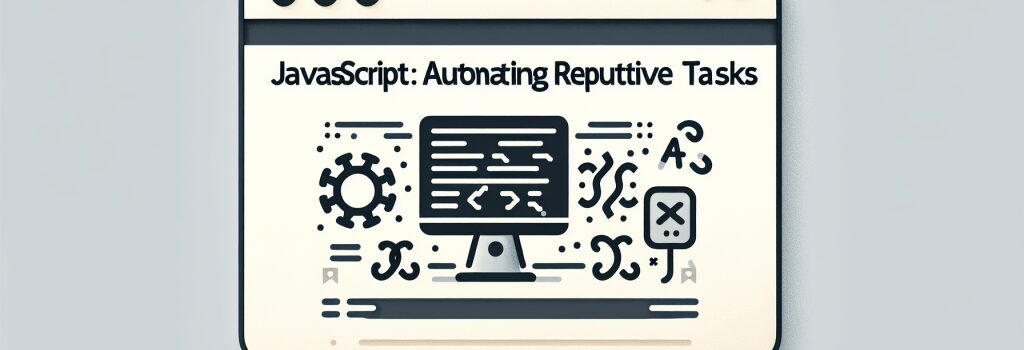
In the realm of web development, mastering JavaScript is akin to acquiring a superpower. It allows you to bring static pages to life, creating interactive and dynamic user experiences. One of the core concepts that you’ll come across in JavaScript is loops – a powerful feature that automates repetitive tasks, making your code more efficient and your programs more effective. This article delves into the various types of JavaScript loops and demonstrates how they can be used to streamline your code.
Understanding JavaScript Loops
Loops, in programming, are constructs that allow you to execute a block of code multiple times without having to write the code repeatedly. This not only saves time but also makes your code cleaner and easier to maintain. JavaScript provides several types of loops, each with its unique use case.
The For Loop
The ;for> loop is perhaps the most common loop you’ll encounter. It is used when you know in advance how many times you need to execute a block of code.
Syntax:
Example:
This will output the numbers 0 to 4 in the console, demonstrating how the loop iterates over the block of code five times, each time incrementing the value of ;i>.
The While Loop
The ;while> loop is ideal for situations where you want to execute a block of code as long as a certain condition is true, but you don’t know how many times the execution should occur.
Syntax:
Example:
Like the ;for> loop example, this will also output numbers 0 to 4. However, the difference lies in how the iteration is controlled.
The Do-While Loop
The ;do-while> loop is a variant of the ;while> loop which ensures that the code block is executed at least once before the condition is tested.
Syntax:
Example:
Even if the condition fails the first test, the ;do-while> loop executes the block of code once, proving useful in certain scenarios.
The For…In Loop
The ;for…in> loop is designed for iterating over the properties of an object.
Syntax:
Example:
This loop will iterate over each property in the ;person> object and display the key-value pairs.
The For…Of Loop
Introduced in ES6, the ;for…of> loop allows you to iterate over iterable objects such as arrays, strings, maps, nodeLists, and more, directly accessing their values.
Syntax:
Example:
This succinctly outputs each color in the array to the console.
Conclusion
JavaScript loops are a fundamental concept that can greatly simplify the process of performing repetitive tasks. By understanding and utilizing the different types of loops provided by JavaScript, developers can write more concise and effective code. Whether you’re iterating through arrays, objects, or simply running a block of code multiple times, loops offer the flexibility and power to meet your coding needs. Happy coding!