JavaScript Events: Interacting with the User
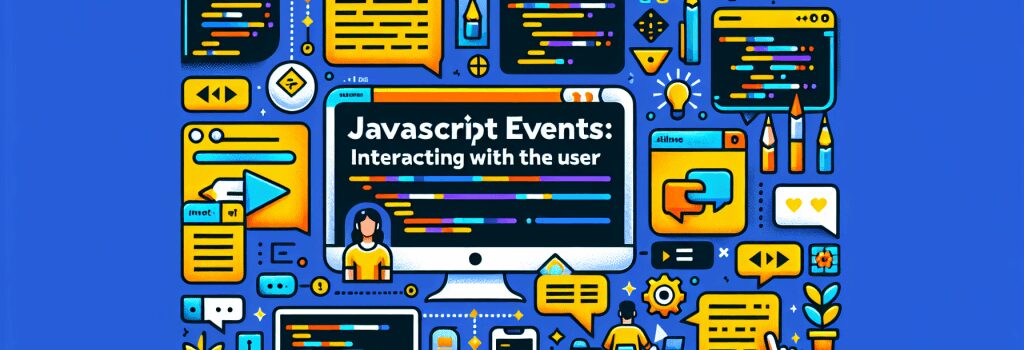
—Understanding the power of JavaScript events is like unlocking a new realm of interactivity for web applications. Events in JavaScript are actions or occurrences that happen in the system you are programming for, such as a website. These can be anything from a simple click to more complex gestures such as swiping or tilting. Grasping how to handle these events is crucial for creating dynamic, responsive, and engaging web applications. This guide delves into the world of JavaScript events, shedding light on how they work and how you can use them to elevate user interaction on your website.
What Are JavaScript Events?
JavaScript events are the browser’s way of signaling that something has happened. This "something" could be a user action like a mouse click, a keypress, touch gestures, or even system-generated events such as a page loading. When these events occur, JavaScript provides a way to execute code in response, allowing web developers to interactively respond to user actions.
Common Types of JavaScript Events
There are numerous events within the JavaScript universe, each with its own specific trigger and use case. Some of the most commonly used JavaScript events include:
– ;click>: Fired when an element is clicked.
– ;load>: Occurs when a page or an image has completely loaded.
– ;mouseover>: Triggered when the mouse pointer moves over an element.
– ;mouseout>: Activated when the mouse pointer moves out of an element.
– ;keydown>: Fired when a key is pressed down.
– ;keyup>: Occurs when a key is released.
Adding Event Listeners
At the heart of utilizing JavaScript events is the concept of event listeners. An event listener is a procedure in JavaScript that waits for an event to occur. You can attach an event listener to any DOM object, not just HTML elements. The syntax for adding an event listener is as follows:
– The ;element> is the target DOM object.
– The ;event> is the type of event you want to listen for.
– The ;function> is the handler that responds to the event.
– The ;useCapture> is a boolean value specifying whether to use capture.
Example: Listening to a Click Event
This code listens for a click event on an element with the id "myButton" and displays an alert when the button is clicked.
Event Propagation: Capturing and Bubbling
Understanding the concepts of event capturing and bubbling is vital in mastering JavaScript events. Event propagation has two phases:
1. Capturing phase: The event goes down to the element.
2. Bubbling phase: The event bubbles up from the element to the root.
By default, event listeners are executed in the bubbling phase. However, setting the ;useCapture> parameter to ;true> in the ;addEventListener()> method lets you run the event handler in the capturing phase instead.
Preventing Default Behavior and Stopping Propagation
Sometimes, you need to prevent the default behavior that belongs to the event or stop the event from propagating along the DOM. JavaScript offers ;preventDefault()> and ;stopPropagation()> methods for this purpose.
Example: Preventing a Form Submission
Conclusion
JavaScript events are a cornerstone of interactive web development, allowing developers to create a dynamic user experience by responding to user actions in real time. By leveraging event listeners, understanding event propagation, and mastering the control of default actions and propagation, developers can craft sophisticated web applications that engage and retain users.
Harnessing the power of JavaScript events opens a plethora of opportunities for creating immersive web experiences. As you continue your journey as a web developer, deepening your understanding and application of JavaScript events will be instrumental in your success.
—