Events and Event Listeners in JavaScript: An Introduction
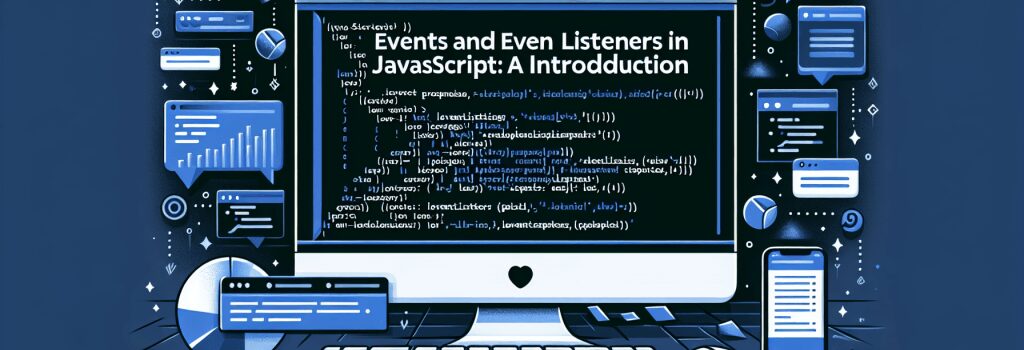
Events and Event Listeners in JavaScript play a pivotal role in creating interactive web applications. They enable web pages to respond to user actions like clicks, key presses, and mouse movements. Understanding how to work with these features is crucial for anyone looking to excel in web development. This article introduces the concept of events and event listeners in JavaScript, providing a foundational understanding for aspiring web developers.
Understanding Events in JavaScript
An event in JavaScript can be described as a significant occurrence that the web browser can detect. This could be anything from a simple mouse click to the browser finishing loading a web page. Events are central to creating dynamic and interactive web applications. They act as a bridge between the user and the system, allowing websites to respond to various user inputs.
Types of Events
JavaScript supports various types of events, including, but not limited to:
– ;click>: Occurs when an element is clicked.
– ;mouseover>: Triggered when the mouse pointer moves over an element.
– ;keydown>: Occurs when a key is pressed down.
– ;load>: Fired when the browser has finished loading the page.
Each of these events offers different functionalities, enabling developers to create complex interactive patterns based on user interaction.
Event Listeners: Listening for Events
Event listeners are crucial in JavaScript as they "listen" for events and execute code in response. Essentially, an event listener waits for a specified event to occur on a target element and then executes a function when that event occurs.
Adding Event Listeners
The ;addEventListener()> method is commonly used to attach an event listener to an element. It takes at least two arguments: the name of the event and the function to be called when the event occurs.
This method allows for greater flexibility and control, enabling developers to easily add and remove event listeners, as well as listen for custom events.
The Event Object
When an event occurs, an event object is automatically passed to the event handler function. This object contains all the details about the event, including the type of event, the target element, and other event-specific information. Developers can leverage this data to create more dynamic and responsive event handlers.
Best Practices for Working with Events and Event Listeners
While working with events and event listeners, following best practices is essential to ensure code maintainability and performance:
1. Use Event Delegation: Instead of attaching event listeners to multiple elements, attach a single event listener to a parent element. This approach is more efficient and reduces memory consumption.
2. Remove Event Listeners: Always remove event listeners when they are no longer needed to prevent memory leaks.
3. Keep Event Handler Functions Light: Ensure that the functions called by event listeners are not overly complex or heavy on computations. This helps in keeping the web application responsive.
4. Use Named Functions: Instead of inline functions, use named functions for event listeners. This makes it easier to remove the event listener when needed.
The understanding and skilled manipulation of events and event listeners form the backbone of interactive web development. For web developers, mastering these concepts is critical in building responsive, user-friendly applications. By grasively leveraging the power of JavaScript events and event listeners, developers can create rich, interactive experiences that engage and retain users.