Declaring and Using Variables in JavaScript Projects
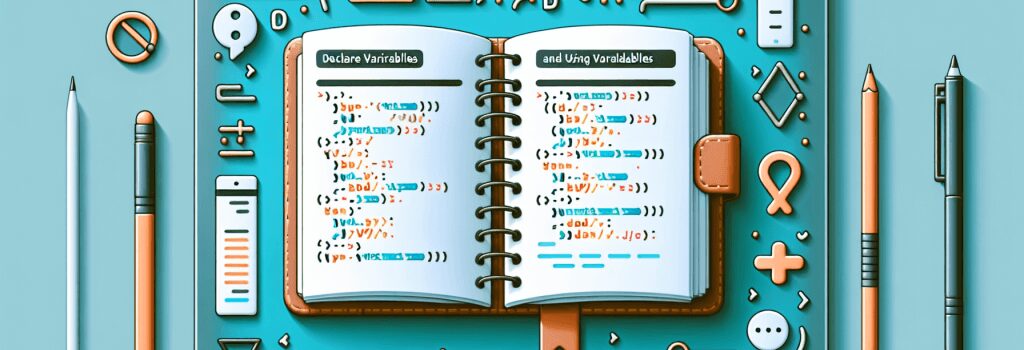
Understanding Variables in JavaScript
Variables are the bread and butter of programming, and JavaScript is no different. They allow developers to store, manipulate, and retrieve data throughout their code. Understanding how to declare and effectively use variables is a foundational skill for any aspiring JavaScript developer. Let’s dive into the world of variables in JavaScript and explore how to leverage them in your projects.
Declaring Variables in JavaScript
In JavaScript, variables can be declared using three keywords: ;var>, ;let>, and ;const>. Each of these has its own scope and usage, which are crucial to understand for writing efficient and error-free code.
Using ;var>
;var> is the oldest keyword used for variable declaration in JavaScript. It defines a variable globally or locally to an entire function regardless of block scope. However, due to its function scope and hoisting nature, it’s less used in modern JavaScript projects.Embracing ;let>
;let> allows you to declare variables that are limited in scope to the block, statement, or expression where they are used. This is more predictable and generally preferred in contemporary coding practices.The Constant ;const>
;const> is used to declare variables whose values are not intended to change. ;const> variables have block scope similar to ;let>. It’s a best practice to use ;const> by default, only using ;let> when you know the variable’s value will change.Working with Data Types and Variables
JavaScript variables can hold many data types: numbers, strings, objects, functions, and more. Understanding the different data types and how to manipulate them is crucial.
Strings and Numbers
Strings are sequences of characters, while numbers can be integers or decimals. JavaScript dynamically handles data types, making it flexible but also tricky.
Objects and Arrays
JavaScript objects are collections of properties, and arrays are a type of object used for storing multiple values in a single variable.
Operators in JavaScript
Operators are symbols that tell the compiler or interpreter to perform specific mathematical, relational, or logical operations and produce a result.
Assignment Operators
Assignment operators, like ;=>, ;+=>, ;-=>, assign values to JavaScript variables.
Arithmetic Operators
Arithmetic operators are used to perform arithmetic on numbers:
Comparison and Logical Operators
These operators are used for conditional statements and include operators like ;==>, ;!=>, ;>>, ;<>, ;&&>, and ;||>.
Best Practices for Using Variables in JavaScript
– Use ;const> by default, only using ;let> when you know the variable’s value will need to change.
– Choose meaningful and descriptive names for variables.
– Stick to lowerCamelCase naming convention for variable names.
– Keep variable declarations at the top of their scope for readability.
Conclusion
Mastering the declaration and use of variables is a fundamental step in becoming proficient in JavaScript. By understanding the different types of variables (;var>, ;let>, ;const>), data types, and operators in JavaScript, developers can write clearer, more efficient code. As you continue to build your skills, keep experimenting with variables in different scenarios to deepen your understanding and application of these concepts in real-world projects.