Custom HTML Elements with JavaScript and the DOM
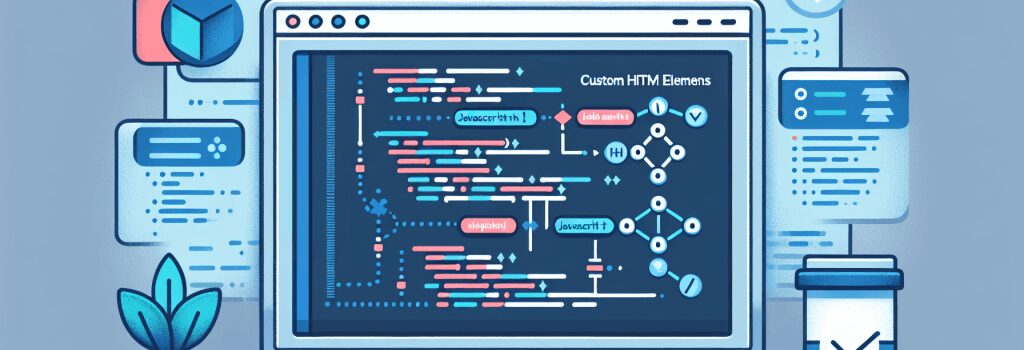
Creating custom HTML elements using JavaScript and the DOM not only allows us to manipulate the existing HTML elements in a web page, but also gives us the power to create new elements, assign dynamic properties to them, and insert them into the web page. It’s a key concept under JavaScript foundations and important for aspiring web developers.
JavaScript and DOM Manipulation
JavaScript is an essential part of the web development stack. It is used to interact with the HTML structure of a web page through an API known as the Document Object Model or DOM. The DOM represents the structure of an HTML page in a tree-like format and allows developers to interact with, alter, and update web page content dynamically.
Creating Custom HTML Elements with JavaScript
To create custom HTML elements with JavaScript, you will need to understand and use methods such as ;createElement()>, ;createTextNode()>, ;setAttribute()>, and ;appendChild()>.
Create Element
The first step is to use the ;createElement()> method to create an HTML element. For example, if you want to create a new div tag, you will write: ;let divElement = document.createElement(‘div’);>.
Assign Properties
Afterwards, you can assign properties to this created element using the ;setAttribute()> method. For example, ;divElement.setAttribute(‘class’, ‘new-div’);>.
Create Text Nodes
If you have a need, you can also create a text node using the ;createTextNode()> method and append it to the new HTML element like this: ;let divText = document.createTextNode(‘Hello World’);> and ;divElement.appendChild(divText);>.
Append to DOM
Finally, append the new HTML element to an existing element in DOM using the ;appendChild()> method. You can append it, for example, to a body element like this: ;document.body.appendChild(divElement);>.
This process will successfully create a new div element with the class ;new-div> and text ;Hello World> then append it to the body part of your HTML document.
Custom HTML Elements in WordPress
WordPress, built mainly in PHP, also allows you to inject custom HTML elements into the webpage with their built-in functions. However, WordPress developers will also need JavaScript and the DOM to handle dynamic and interactive changes.
Conclusion
Creating custom HTML elements extends the design and functionality of web pages. With JavaScript and the DOM, the website designs are not bound by static HTML alone. As a result, you can program dynamic and interactive features providing value and enhanced user experience. Understanding how to create and manipulate DOM elements with JavaScript is a critical part of becoming a successful web developer.