Creating and Appending Elements Dynamically with JavaScript
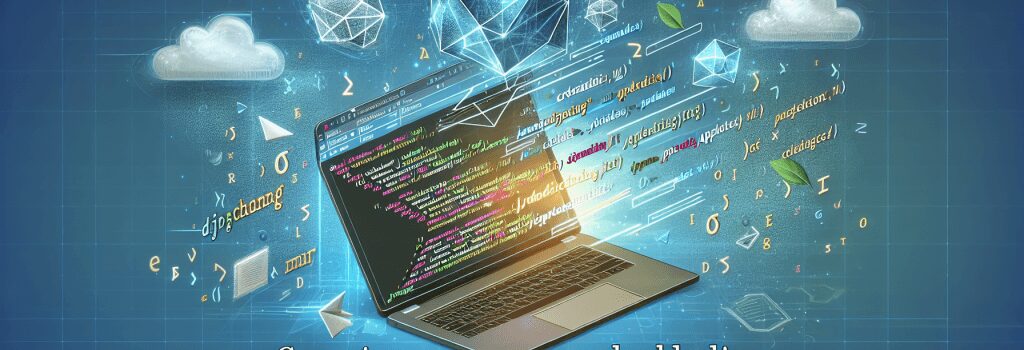
Understanding Dynamic Element Creation in JavaScript
In the world of web development, JavaScript stands out as a powerful tool that enhances interactivity and user experience on web pages. One of the essential skills for a web developer is manipulating the Document Object Model (DOM) using JavaScript. This involves creating, modifying, and deleting elements on a webpage dynamically without needing to reload the page. This article delves into how to dynamically create and append elements in the DOM using JavaScript, an indispensable technique for modern web development.
The Basics of the Document Object Model (DOM)
Before we jump into creating and appending elements, let’s briefly review what the DOM is. The DOM is a programming interface that allows scripts to update the content, structure, and style of a document. Essentially, it represents the page so that programs can change the document structure, style, and content. The DOM represents the document as a tree of objects; each element is a node in this tree.
Creating Elements Dynamically
JavaScript offers a straightforward method to create elements dynamically: the ;document.createElement()> method. This method creates an HTML element specified by a tag name as its parameter. Here’s a simple example:
This code snippet creates a new ;<div>> element. However, this new element does not appear on the webpage yet because it has not been inserted into the DOM tree.
Setting Attributes and Content
After creating an element, you often need to set its attributes or inner content. This can be done using the ;setAttribute()> method and the ;textContent> property, respectively. Here’s how:
In this example, we’ve added a class attribute to our ;<div>> and also provided some text content.
Appending Elements to the DOM
To have the newly created element appear on the webpage, you need to append it to an existing element within the DOM tree. This is typically done using the ;appendChild()> method. Determine where you want to place your new element and append it as a child. For instance, to append it to the body of the document:
Now, the ;<div>> element we created dynamically with JavaScript will appear on the page.
Inserting Elements at Specific Positions
Sometimes, appending an element as the last child isn’t what you want. JavaScript provides methods like ;insertBefore()> for more control over the positioning of dynamically created elements. For instance:
This example inserts the new element as the first child of the identified parent element, rather than appending it to the end.
Conclusion
Dynamically creating and appending elements with JavaScript is a fundamental technique in web development, enabling developers to build highly interactive and responsive web applications. Mastering DOM manipulation allows for the dynamic addition and modification of content, enhancing the user experience without reloading the page. Practice these skills to become proficient in manipulating web pages in real-time, making your web applications more dynamic and engaging for users.
By understanding how to use ;document.createElement()>, setting attributes and content, and properly appending elements to the DOM, you’re well on your way to unlocking the full potential of JavaScript in web development.