Advanced DOM Manipulation: Beyond the Basics
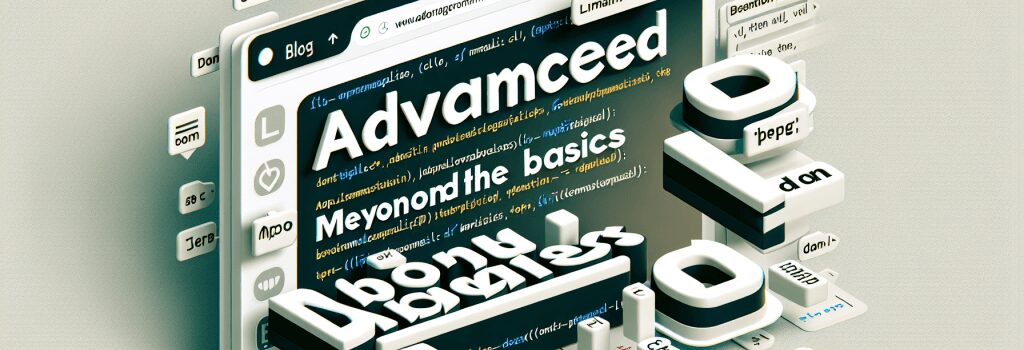
Understanding and manipulating the Document Object Model (DOM) is a vital skill for web developers aiming to create interactive and dynamic web applications. Beyond the basic operations like selecting, modifying, adding, and removing elements, advanced DOM manipulation techniques allow for more sophisticated interactions and enhanced web performance. This article delves into these heightened techniques, providing a comprehensive guide to elevating your web development skills.
Advanced Techniques in DOM Manipulation
The Power of Node Cloning
Node cloning is an advanced technique that allows developers to duplicate nodes within the DOM. This is particularly useful when you need to create copies of elements without having to recreate them from scratch. The ;cloneNode()> method can be used, where passing ;true> as an argument clones the node and its entire subtree, and ;false> clones only the node.
Mastering Document Fragments
Document fragments represent a minimal document object without a parent in the DOM tree. They serve as lightweight containers used to hold temporary DOM elements. Working with document fragments can significantly improve performance when manipulating or adding multiple DOM elements. By appending elements to the fragment and then appending the fragment to the document, you minimize the number of reflows and repaints, enhancing your application’s efficiency.
Event Delegation and Propagation
Understanding event delegation and propagation is crucial in managing complex event handling scenarios. Event delegation allows for the binding of a single event listener to a parent element rather than multiple listeners to individual child elements. This not only simplifies event management but also conserves memory. It’s essential to grasp the concepts of event bubbling and capturing to effectively control event propagation throughout the DOM.
Utilizing the MutationObserver API
The MutationObserver API provides the ability to watch for changes being made to the DOM tree. This is particularly helpful in developing applications that rely on dynamic content. By creating a mutation observer, developers can specify which DOM mutations to monitor and react programmatically to changes in the DOM structure, attributes, or content. This allows for creating highly responsive and adaptive web applications.
Optimizing DOM Manipulation for Performance
Minimizing DOM Access
Direct DOM manipulation is an expensive operation in terms of performance. To enhance efficiency, it’s advisable to minimize the frequency of DOM access. This can be achieved by caching references to DOM elements and avoiding unnecessary DOM queries.
Debouncing and Throttling
Debouncing and throttling are two techniques aimed at controlling the rate at which certain operations are performed. These are particularly useful in handling events that can fire frequently, such as window resizing or scroll events. Debouncing ensures that a function is executed after a certain amount of time has elapsed since the last time it was invoked, while throttling limits the execution of a function to no more than once in a specified time frame.
Conclusion
Mastering advanced DOM manipulation techniques is a stepping stone towards becoming a proficient web developer. By understanding and implementing these strategies, you can create more efficient, dynamic, and responsive web applications. Embracing these practices will not only improve your coding skills but also enhance the user experience of the websites and applications you develop. Remember, the key to successful DOM manipulation lies in practicing and applying these techniques in real-world projects.