Implementing Form Validation: Client-Side Techniques
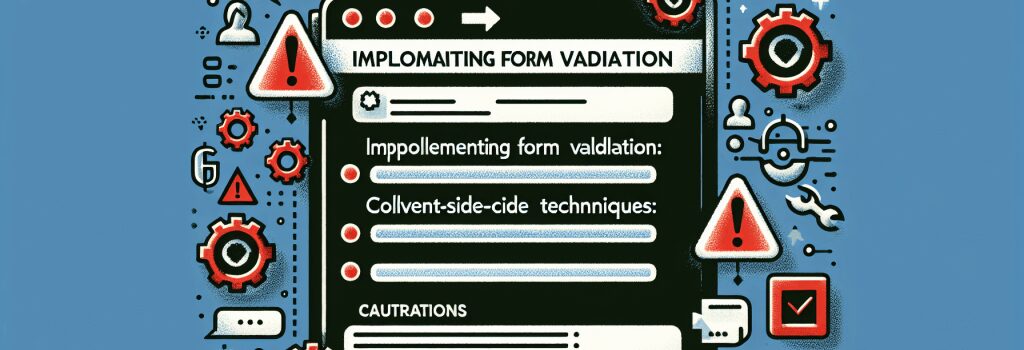
Introduction to Form Validation
In the world of web development, form validation plays a crucial role in ensuring the integrity and security of data submitted by users. It’s a process where you check if the information provided by the user meets certain criteria before you allow it to be processed or stored. While server-side validation is essential for security, client-side validation enhances user experience by providing immediate feedback. In this article, we’ll delve into implementing client-side form validation techniques using HTML and JavaScript.
Understanding Client-Side Validation
Client-side validation is performed in the user’s browser before the data is sent to the server. This immediate feedback can prevent unnecessary server load and enhance the user experience by catching errors early.HTML5 Validation Features
HTML5 introduced several attributes that make implementing basic validation straightforward and without the need for JavaScript. Attributes such as ;required>, ;type>, ;min>, ;max>, and ;pattern> allow developers to define acceptable input criteria directly in the HTML markup.
– The ;required> attribute specifies that an input field cannot be left empty.
– The ;type> attribute specifies the type of data expected (e.g., email, number), and browsers can automatically validate the input based on it.
– The ;min> and ;max> attributes define the permissible range of values for numeric inputs.
– The ;pattern> attribute allows defining a regular expression against which the input value is checked.
Enhancing Validation with JavaScript
While HTML5 validation is powerful, JavaScript offers more flexibility and control over the validation process. You can respond to various events, such as ;onsubmit> for form submission or ;onchange> for individual inputs, to perform validation.
Implementing JavaScript Validation
1. Capturing User InputFirst, access the form element and inputs within it. This can be done using ;document.getElementById> or ;document.querySelector>.
2. Listening for Form Submission
Attach an event listener to the form’s ;submit> event. Within the event handler, prevent the form’s default submission initially, to validate the input fields first.
3. Validating Inputs
Create a ;validateForm> function where you define the validation logic. For example, checking if an email field is empty or doesn’t match a regular expression pattern for email addresses.
4. Providing Feedback
Provide immediate visual feedback for validation errors. This might involve displaying error messages or changing the appearance of invalid inputs (e.g., border color).
Conclusion
Client-side validation is an integral part of creating responsive and user-friendly web forms. By combining HTML5 validation features with the power of JavaScript, developers can ensure a robust validation mechanism that enhances user experience and prevents the submission of incorrect data. Remember, while client-side validation improves usability, it does not replace the need for server-side validation for security reasons.