PHP Error Handling: Techniques for a Robust Website
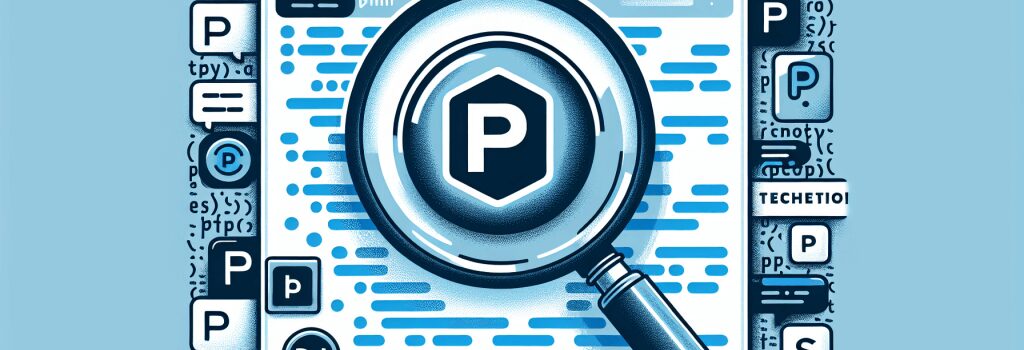
Understanding PHP Error Handling
Error handling in PHP is a critical skill for web developers aiming to create robust and reliable websites. With the correct techniques, developers can prevent their websites from crashing and provide a smooth user experience. This article explores essential PHP error handling methods to enhance your web development skills.
Types of Errors in PHP
Before diving into error handling techniques, it’s essential to understand the types of errors you might encounter in PHP:
1. Syntax Errors: These occur when there’s a typo or mistake in the code that prevents the script from running.
2. Runtime Errors: These happen during the execution of the script and can stop the script from completing.
3. Logical Errors: Often the hardest to detect and fix, these errors occur when the script runs without crashing but produces incorrect results.
Basic PHP Error Handling Techniques
Using ;die()> and ;exit()>
The simplest way to handle errors in PHP is by using the ;die()> or ;exit()> functions. These functions stop the execution of the script immediately after an error is encountered, optionally allowing you to output a custom error message.
php
if (!file_exists("example.txt")) {
die("File not found.");
} else {
$file = fopen("example.txt", "r");
}
While this method is straightforward, it’s not always the most user-friendly approach, as it halts the entire script abruptly.
Custom Error Handlers with ;set_error_handler()>
For a more refined error handling process, PHP allows you to define a custom error handler function. This function can be used to log errors, send notification emails, or display user-friendly error messages without stopping the script.
php
function customError($error_level, $error_message, $error_file, $error_line) {
echo "<b>Error:</b> [$error_level] $error_message<br>";
echo "Location: $error_file on line $error_line<br>";
}
set_error_handler("customError");
Advanced PHP Error Handling Techniques
Exception Handling with Try-Catch Blocks
PHP supports exception handling using try-catch blocks, providing a sophisticated approach to managing errors. Exceptions are used to signal errors that are not always fatal, allowing the script to continue running with proper error handling routines.
php
try {
// Code that may throw an exception
throw new Exception("An error occurred.");
} catch (Exception $e) {
// Handle exception
echo "Error: " . $e->getMessage();
}
Error Reporting Levels
PHP allows you to define different levels of error reporting to control which errors are reported and handled. This is useful during development and production stages, as you may want more detailed error reporting while developing and less verbose reporting in production.
php
error_reporting(E_ALL); // Report all errors
error_reporting(E_ERROR | E_WARNING); // Report only errors and warnings
error_reporting(0); // Turn off all error reporting
Best Practices for PHP Error Handling
– Log Errors for Further Analysis: Always log errors to a file or database for further analysis. This can help identify recurring issues and areas for improvement.
– Display User-Friendly Error Messages: Never expose raw error messages to users. Instead, display helpful and user-friendly messages.
– Develop With All Error Reporting Enabled: During development, enable all error reporting to catch and fix as many issues as possible.
– Use Exceptions for Object-Oriented Programming: When working with object-oriented PHP, use exceptions to handle errors within classes and methods.
Conclusion
Mastering error handling in PHP is fundamental to building reliable and user-friendly websites. By understanding the different types of errors and implementing sophisticated error handling techniques like custom error handlers and exception handling, you can significantly improve the quality of your web applications. Remember, the goal of error handling is not just to prevent crashes, but also to create a seamless experience for the user, regardless of any underlying issues.