JavaScript Challenges: Preparing for Your Web Development Interview
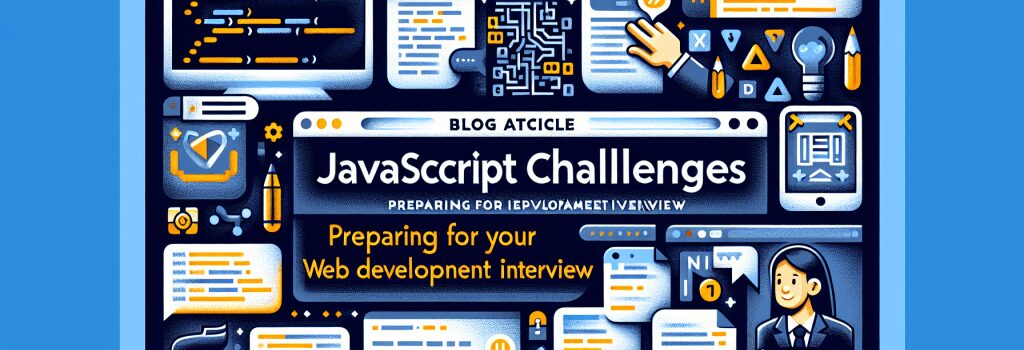
Introduction to JavaScript Challenges in Web Development Interviews
As you advance in your journey to becoming a skilled web developer, mastering JavaScript is crucial. It’s not just about knowing the syntax and how to manipulate the DOM. Preparing for web development interviews means being ready to face JavaScript challenges that test your problem-solving skills, understanding of the language, and ability to apply concepts in real-world scenarios.
Understanding the Role of JavaScript in Web Development Interviews
JavaScript is an essential part of the web development stack. It breathes life into websites, making them interactive and functional. As such, interviewers use JavaScript challenges to gauge a candidate’s technical prowess and their problem-solving approach.
Types of JavaScript Challenges
Expect to encounter a range of challenges that test various JavaScript concepts such as:
– Variable Scoping and Hoisting: Understanding how variables are scoped and hoisted in JavaScript can be pivotal in writing efficient and bug-free code.
– Asynchronous Programming: Challenges might include working with promises, async/await, or callbacks to handle asynchronous operations.
– DOM Manipulation: Since JavaScript is used to enhance user interfaces, expect tasks requiring you to dynamically change the DOM.
– Event Handling: Reacting to user inputs by creating and managing events is a common challenge.
– Algorithmic Questions: Common coding interview challenges that test your logical thinking and problem-solving skills with algorithms.
Preparing for JavaScript Challenges
Brush Up on JavaScript Fundamentals
Ensure you’re comfortable with JavaScript fundamentals. This includes understanding primitive types, variable declarations (;let>, ;const>, ;var>), functions (including arrow functions), and more complex topics like closures, hoisting, and the prototype chain.
Practice Common Algorithm Challenges
Many interviews include algorithmic challenges. Practice with common data structures (arrays, objects) and algorithms (sorting, searching) to enhance your problem-solving skills.
Understand Asynchronous JavaScript
Asynchronous JavaScript is a critical part of modern web development. Familiarize yourself with callbacks, promises, and async/await. Understand how the JavaScript event loop works and how to handle asynchronous operations.
Get Comfortable with DOM Manipulation and Event Handling
Create small projects or exercises where you dynamically update the DOM based on user input or events. This will help you get comfortable with common tasks like creating, reading, updating, or deleting elements on a webpage.
Mock Interviews
Practice makes perfect. Participate in mock interviews with peers or use online platforms to simulate the interview environment. This can help reduce anxiety and improve your problem-solving speed under pressure.
JavaScript Coding Challenge Examples
To give you a head start, here are some examples of challenges you might face:
1. Palindrome Checker: Write a function that checks if a string is a palindrome.
2. Todo List: Create a simple todo list where users can add tasks, mark them as completed, and delete them.
3. Weather App: Fetch weather data from a public API and display it dynamically on a webpage.
Conclusion
JavaScript challenges in web development interviews can range from simple algorithmic questions to tasks that simulate real-world web development scenarios. Preparing for these challenges requires a solid understanding of JavaScript fundamentals, practical coding experience, and continuous practice with mock interviews. By following these guidelines, you’ll be well-equipped to tackle any JavaScript challenge thrown your way during your web development interviews.