Working with Variables and Data Types in PHP
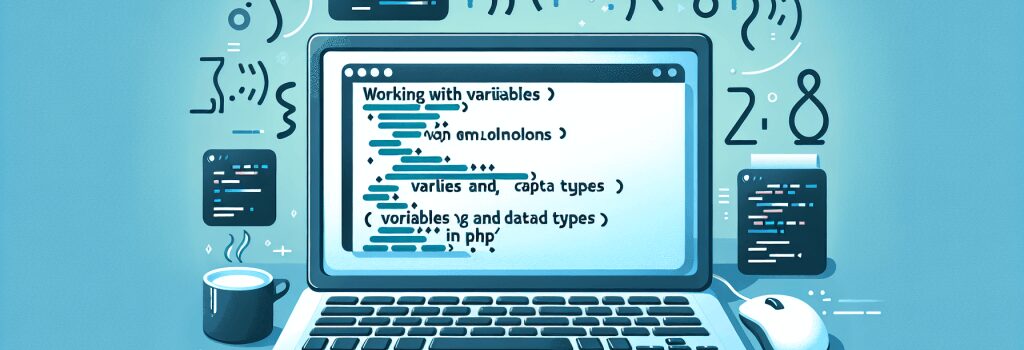
Understanding Variables and Data Types in PHP
PHP, a powerful server-side scripting language, is a foundational tool for many web developers. In this segment, we’ll delve into the basics of working with variables and data types in PHP, an essential skill for anyone aiming to master backend development. By understanding these concepts, you’ll be able to store, manipulate, and retrieve data efficiently, setting a strong base for your coding projects.
What are Variables in PHP?
Variables in PHP are used to store data that can be used and manipulated throughout your code. They are identifiers that allow you to hold and manage values within a PHP script. In PHP, a variable starts with the ;$> symbol, followed by the name of the variable. The naming of these variables must start with a letter or an underscore, not a number, and they are case-sensitive.
Declaring Variables
To declare a variable in PHP, you simply write the ;$> symbol followed by the variable name and assign it a value using the ;=> operator. Here’s an example:
In this line, ;$greeting> is the variable, and ;"Hello, World!"> is the value assigned to it.
Data Types in PHP
PHP supports various data types that determine the nature of the value a variable can hold. These data types are categorized into three main groups: scalar types, compound types, and special types.
Scalar Types
Scalar types are simple, single-value types. They include:
– Integer: A non-decimal number between -2^31 and 2^31-1 in 32-bit systems. For example, ;$age = 25;>.
– Float (or double): A number with a decimal point or a number in exponential form. For example, ;$price = 10.99;>.
– String: A sequence of characters inside quotes. It can be either single-quoted ;’Hello’> or double-quoted ;"Hello">. For example, ;$name = "John Doe";>.
– Boolean: Represents two possible states: ;TRUE> or ;FALSE>. For example, ;$is_logged_in = FALSE;>.
Compound Types
Compound types can hold multiple values and include:
– Array: An ordered map of values. For example, ;$fruits = array("apple", "orange", "banana");>.
– Object: An instance of a class. You can define a class and then create an object of that class. For example:
Special Types
Special types include resources and NULL:
– Resource: A special variable that holds a reference to resources outside of PHP (like a database connection).
– NULL: Represents a variable with no value. For example, ;$a = NULL;>.
Understanding and using these data types effectively will enable you to handle a variety of data manipulation and storage tasks in your PHP code.
Conclusion
Variables and data types are core concepts in PHP programming, crucial for data storage and manipulation. By grasping these fundamental topics, you’re well on your way to becoming proficient in backend development with PHP. Remember, practice is key to mastering these concepts, so experiment with different types of data and variable declarations to see first-hand how they work.