Using Conditional Operators in PHP for Effective Decision Making
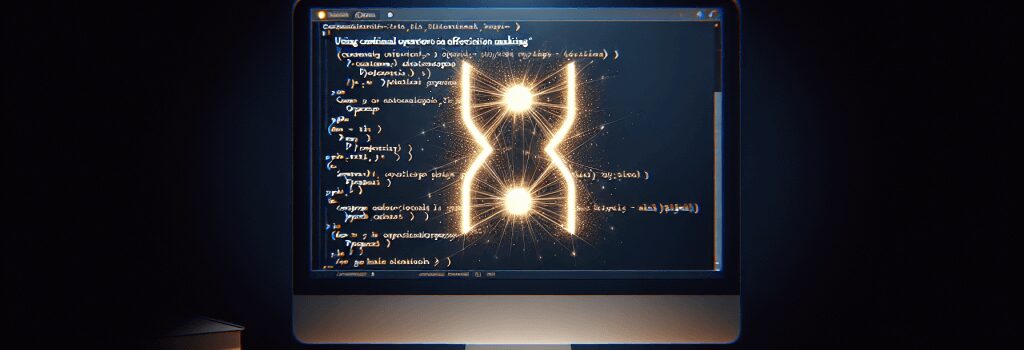
Using Conditional Operators in PHP for Effective Decision Making
Introduction to Conditional Operators in PHP
Conditional operators in PHP are the backbone of decision-making processes within your code. They allow your program to execute different code blocks based on certain conditions. Understanding how to use these operators effectively can greatly enhance your application’s logic and performance.
Understanding the Basics of Conditional Operators
Conditional operators evaluate an expression to produce a result that is either true or false. PHP supports several conditional operators, including:
– If Statement: The most basic form of conditional operator. It executes a block of code if the specified condition is true.
– If-else Statement: Extends the functionality of an if statement by adding an alternative action if the condition is false.
– Elseif Statement: Allows you to specify a new condition if the first condition is false.
– Switch Statement: A more efficient alternative when you have multiple conditions to check.
– Ternary Operator: A shorthand for the if-else statement, allowing you to execute code blocks inline.
Advantages of Using Conditional Operators
Implementing conditional operators in your PHP scripts provides several benefits:
– Improved Readability: Clear usage of conditional operators makes your code easier to read and maintain.
– Efficient Coding Practice: Helps in writing concise and less cluttered code, especially with the ternary operator.
– Better Logic Handling: Enhances your application’s logic flow, making it more flexible and dynamic.
Implementing Conditional Operators in PHP
The If Statement
This simple if statement checks if the age is greater than 18. If the condition is true, it prints a message.
The If-else Statement
If-else statements provide an alternative action if the condition fails.
The Elseif Statement
Elseif statements allow you to check multiple conditions sequentially.
The Switch Statement
Switch statements are beneficial when you have multiple distinct values to check against a single variable.
The Ternary Operator
The ternary operator offers a compact way to write simple if-else conditions.
Conclusion
Mastering the use of conditional operators in PHP is crucial for any aspiring web developer. They not only make your code more readable and maintainable but also empower you to build sophisticated and dynamic web applications. By understanding and implementing these operators, you can create more efficient and powerful PHP scripts tailored to your application’s specific needs. Remember, practice is key to getting comfortable with these conditional operators. Keep experimenting with different scenarios, and you’ll find them becoming an integral part of your PHP programming toolkit.